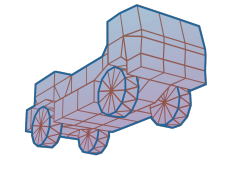 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
37 #define CONFIG_FILE_NAME "RoR.cfg"
96 else {
return GfxShadowType::NONE ; }
103 else {
return GfxExtCamMode::NONE ; }
112 else {
return GfxTexFilter::NONE ; }
121 else {
return GfxVegetation::NONE ; }
131 else {
return SimGearboxMode::AUTO ; }
141 else {
return GfxFlaresMode::CURR_VEHICLE_HEAD_ONLY ; }
152 else {
return GfxWaterMode::BASIC ; }
160 else {
return GfxSkyMode::SANDSTORM ; }
283 int rate = Ogre::StringConverter::parseInt(val);
284 if (rate < 0) { rate = 0; }
285 if (rate > 6) { rate = 6; }
290 int quality = Ogre::StringConverter::parseInt(val);
291 if (quality < 0) { quality = 0; }
292 if (quality > 3) { quality = 3; }
330 int fov = Ogre::StringConverter::parseInt(val);
342 void Console::loadConfig()
344 Ogre::ConfigFile cfg;
348 cfg.load(path,
"=:\t",
true);
350 Ogre::ConfigFile::SettingsIterator i = cfg.getSettingsIterator();
351 while (i.hasMoreElements())
372 catch (Ogre::FileNotFoundException&) {}
377 f << std::endl <<
"; " << label << std::endl;
381 if (pair.second->hasFlag(
CVAR_ARCHIVE) && pair.first.find(prefix) == 0)
385 f << pair.second->getLongName() <<
"=";
389 f << pair.second->getName() <<
"=";
400 else { f << pair.second->getStr(); }
407 void Console::saveConfig()
411 f <<
"; Rigs of Rods configuration file" << std::endl;
412 f <<
"; -------------------------------" << std::endl;
425 Ogre::DataStreamPtr stream
426 = Ogre::ResourceGroupManager::getSingleton().createResource(
428 size_t written = stream->write(f.str().c_str(), f.str().length());
429 if (written < f.str().length())
431 RoR::LogFormat(
"[RoR] Error writing file '%s' (resource group '%s'), ",
432 "only written %u out of %u bytes!",
436 catch (std::exception& e)
438 RoR::LogFormat(
"[RoR|Settings] Error writing file '%s' (resource group '%s'), message: '%s'",
const char * CONF_EXTCAM_NONE
const char * GfxWaterModeToStr(GfxWaterMode v)
SimGearboxMode ParseSimGearboxMode(std::string const &s)
const char * CONF_VEGET_20PERC
CVar * cVarGet(std::string const &input_name, int flags)
Get cvar by short/long name, or create new one using input as short name.
const char * CONF_FLARES_NO_LIGHT
const char * CONF_GFX_SHADOW_PSSM
const char * GfxSkyModeToStr(GfxSkyMode v)
const char * CONF_WATER_FULL_FAST
const char * CONF_FLARES_NONE
const char * CONF_TEXFILTER_ANISO
const char * IoInputGrabModeToStr(IoInputGrabMode v)
const char * CONF_GEARBOX_MANUAL
const char * CONF_TEXFILTER_TRILI
const char * SimGearboxModeToStr(SimGearboxMode v)
const char * CONF_INPUT_GRAB_NONE
GfxShadowType ParseGfxShadowType(std::string const &s)
const char * CONF_SKY_CAELUM
const char * CONF_TEXFILTER_NONE
std::string SanitizeUtf8String(std::string const &str_in)
CVar * cVarFind(std::string const &input_name)
Find cvar by short/long name.
void LogFormat(const char *format,...)
Improved logging utility. Uses fixed 2Kb buffer.
CVar * gfx_fov_external_default
const char * CONF_WATER_NONE
const char * GfxShadowTypeToStr(GfxShadowType v)
CVar * gfx_texture_filter
const char * CONF_EXTCAM_STATIC
IoInputGrabMode ParseIoInputGrabMode(std::string const &s)
@ CVAR_ARCHIVE
Will be written to RoR.cfg.
GfxExtCamMode ParseGfxExtCamMode(std::string const &s)
const char * CONF_VEGET_50PERC
const char * GfxFlaresModeToStr(GfxFlaresMode v)
const char * CONF_WATER_REFLECT
void AssignHelper(CVar *cvar, int val)
CVar * app_config_long_names
Wrapper for classic c-string (local buffer) Refresher: strlen() excludes '\0' terminator; strncat() A...
void cVarAssign(CVar *cvar, std::string const &value)
Parse value by cvar type.
const char * CONF_INPUT_GRAB_ALL
const char * CONF_TEXFILTER_BILI
const char * CONF_WATER_HYDRAX
std::string PathCombine(std::string a, std::string b)
const char * CONF_SKY_SKYX
const char * CONF_SKY_SANDSTORM
const char * CONF_FLARES_ALL_HEADS
const char * ToCStr() const
const char * CONF_GFX_SHADOW_NONE
GfxWaterMode ParseGfxWaterMode(std::string const &s)
const char * CONF_WATER_BASIC
const char * CONF_FLARES_ALL_LIGHTS
Central state/object manager and communications hub.
bool hasFlag(int f) const
CVar * gfx_fov_internal_default
void WriteVarsHelper(std::stringstream &f, const char *label, const char *prefix)
const char * CONF_GEARBOX_MAN_RANGES
const char * GfxExtCamModeToStr(GfxExtCamMode v)
Quake-style console variable, defined in RoR.cfg or crated via Console UI and scripts.
void ParseHelper(CVar *cvar, std::string const &val)
CVar * gfx_shadow_quality
const char * CONF_INPUT_GRAB_DYNAMIC
const char * CONF_GEARBOX_AUTO
std::string const & getName() const
const char * GfxVegetationToStr(GfxVegetation v)
const char * CONF_EXTCAM_PITCHING
GfxTexFilter ParseGfxTexFilter(std::string const &s)
GfxSkyMode ParseGfxSkyMode(std::string const &s)
const char * CONF_FLARES_CURR_HEAD
const char * GfxTexFilterToStr(GfxTexFilter v)
const char * CONF_WATER_FULL_HQ
CVar * gfx_vegetation_mode
const char * CONF_GEARBOX_SEMIAUTO
const char * CONF_GEARBOX_MAN_STICK
const char * CONF_VEGET_FULL
GfxFlaresMode ParseGfxFlaresMode(std::string const &s)
const char * CONF_VEGET_NONE
GfxVegetation ParseGfxVegetation(std::string const &s)