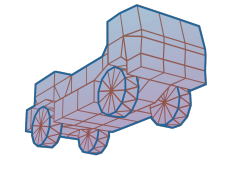 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
35 void raceEvent(
int trigger_type,
string inst,
string box,
int nodeid);
114 void raceEvent(
int trigger_type,
string inst,
string box,
int nodeid);
134 void addLapTime(
int raceID,
double time,
bool &out newBestLap);
136 void addRaceTime(
int raceID,
double time,
bool &out newBestRace);
177 int addRace(
string raceName,
double[][] checkpoints,
int laps,
string objName_checkpoint,
string objName_start,
string objName_finish);
188 int addRace(
string raceName,
double[][] checkpoints,
int laps,
string objName_checkpoint,
string objName_start_finish);
198 int addRace(
string raceName,
double[][] checkpoints,
int laps,
string objName_checkpoint);
207 int addRace(
string raceName,
double[][] checkpoints,
int laps);
215 int addRace(
string raceName,
double[][] checkpoints);
393 void setLaps(
int raceID,
int laps);
432 void addCheckpoint(
int raceID,
int number,
string objName,
double[] coords);
447 void addCheckpointList(
int raceID, uint startNumber,
string objName,
double[][] coords);
604 void addChpCoordinates(
double[][] checkpoints_in,
const string &in objName_checkpoint,
const string &in objName_start,
const string &in objName_finish, uint startNumber);
607 void addCheckpoint(
int number,
const string &in objName,
const double[] &in v);
617 void saveRace(Script2Game::LocalStorage@ d);
618 void loadRace(Script2Game::LocalStorage@ d);
int LAPS_NoLaps
This race has a seperate start line and finish line.
uint getRealInstanceCount(int chpNum)
void setBestRaceTime(int raceID, double time)
Sets a best race time.
void raceEvent(int trigger_type, string inst, string box, int nodeid)
This will get called when a truck is at a checkpoint You shouldn't call this manually (use the "Check...
string raceManagerVersion
the version of the raceManager
bool raceCompleted(int raceID)
Is a certain race completed?
void advanceCheckpoint(int raceID)
Advances a checkpoint.
racesManager()
The constructor This initializes everything.
void advanceLap()
Advances a lap.
int ACTION_SuspendRace
Not used.
int addRace(string raceName, double[][] checkpoints, int laps, string objName_checkpoint, string objName_start, string objName_finish)
Adds a race to the terrain.
void addCheckpointList(int raceID, uint startNumber, string objName, double[][] coords)
Adds a list of checkpoints to a race.
bool addPenaltySeconds(int seconds)
Add an amount of penalty time to the current race.
int ARROW_AUTO
This arrow method will always point to the checkpoint instance that comes closest to the previous one...
void raceCancelPointHandler(int trigger_type, string inst, string box, int nodeid)
called when the user drives through a cancel point
int getRaceIDbyName(string raceName_in)
Returns the race ID of a race name.
void deleteCheckpoint(int number)
string raceBuilderVersion
array< array< string > > objNames
void setupArrow(int position)
Make the directional arrow point to a certain checkpoint of the current race.
void raceCancelPointHandler(int trigger_type, string inst, string box, int nodeid)
event handler for cancel points
void loadRace(Script2Game::LocalStorage@ d)
int STATE_Waiting
A race is suspended, and we're waiting until the race continues.
void resetEventCallback()
Resets the race event callback.
bool abortOnVehicleExit
if true: if the user exits his vehicle, the race will be aborted. (default=false)
funcdef void RACE_EVENT_CALLBACK(dictionary@)
A function signature for the callback pointers.
private raceBuilder[] raceList
string formatTime(double seconds)
Formats the time.
void addRaceTime(int raceID, double time, bool &out newBestRace)
int LAPS_Unlimited
Keep looping the race for an unlimited time.
void lockRace(int raceID)
Locks a race.
void loadRace(int raceID)
Loads a race.
bool showCheckPointInfoWhenNotInRace
if true: if the user drives through a checkpoint of a race that isn't running, a message will be show...
int getPreviousCheckpointNum(int lastCheckpoint)
void addCancelPoint(int raceID, string objName, double[] v, RACE_EVENT_CALLBACK @callback)
This allows you to add an object with event boxes that will cause the race to be aborted.
int addNewEmptyRace()
Add a new empty race (advanced users only!)
void setBestLapTime(int raceID, double time)
Sets a best lap time.
bool silentMode
if true: No flashmessages will be shown. (default=false)
void raceEvent(int trigger_type, string inst, string box, int nodeid)
called when the user drives through a checkpoint
int arrowMethod
What checkpoint instance should the directional arrow point to. (default=ARROW_AUTO=point to the same...
void addCheckpoint(int raceID, int number, string objName, double[] coords)
Adds a checkpoint to a race.
void addLapTime(int raceID, double time, bool &out newBestLap)
void saveRace(Script2Game::LocalStorage@ d)
void eventCallback(int eventnum, int value)
This should be called from within your RoR eventCallback function.
int STATE_NotInRace
We're not racing at the moment.
void setRaceName(int raceID, string raceName)
Sets the name for a race.
bool showBestLap
if true: If a race is started or a new best lap is set, the best lap will be shown....
bool obligatedFinish
if true: if you drive through the start checkpoint of another race, while racing, it will be ignored....
int lastCheckpoint
The number of the last passed checkpoint.
void setLaps(int raceID, int laps)
Sets the amount of laps for a race.
double getBestLapTime(int raceID)
Gets the best lap time of a certain race.
void setLaps(int laps_in)
This class manages a race (singular!) You should only use this directly if the racesManager doesn't s...
void finalize(int raceID)
Finalizes your (advanced) race.
int getNextCheckpointNum(int lastCheckpoint)
void setVersion(const string &in version)
void deleteCheckPoint(int raceID, int number, int instance)
This deletes a checkpoint from a race.
void deleteRace(int raceID)
Deletes a race.
double getBestRaceTime(int raceID)
Gets the best race time of a certain race.
void removeArrow()
This hides the directional arrow.
int STATE_Racing
We're in the middle of a race :D.
int ACTION_DoNothing
Not used.
void setPenaltyTime(int raceID, int seconds)
This sets the penalty time of a race.
Pseudo-namespace; it doesn't exist in code or script runtime, only in this documentation.
bool submitScore
if true: If the user has a new best lap or new best race, this is submitted to the master server....
int getNewRaceID()
Creates a new free race ID.
void removeCallback(string event)
Remove a callback function.
void cancelCurrentRace()
Aborts the current race.
bool showTimeDiff
if true: Show + or - [best time minus current time] when passing a checkpoint. (default=true)
void addCheckpoint(int number, const string &in objName, const double[] &in v)
int getPenaltyTime(int raceID)
This returns the penalty time setting of a race.
raceBuilder getRace(int raceID)
Gets the raceBuilder object of a race.
void unlockRace(int raceID)
Unlocks a race.
int ACTION_RestartRace
Not used.
int getCurrentRaceID()
Gets the ID of the current race.
int raceCount
the raceID+1 of the last race.
double[] lastTimeTillPoint
void setStartNumber(int raceID, int startNum)
This allows you to change the startNumber of a race.
int LAPS_One
This race consist of 1 lap, and the start and finish line are the same object.
int currentLap
the number of the current lap. (starts from 0)
or anywhere else will not be considered a but parsed as regular data ! Each line is treated as values separated by separators Possible i e animators Multiline description Single instance
void saveRaces()
Saves all races.
int ACTION_StopRace
Not used.
void hideRace(int raceID)
This hides your race.
RACE_EVENT_CALLBACK getCallback(string event)
Gets a callback funciton.
void setVersion(int raceID, string version)
This sets the version of your race.
void recalcArrow()
Recalculates where the arrow should point to.
void saveRace(int raceID)
Saves a race.
This class allows you to organize races.
int getLaps(int raceID)
Gets the amount of laps for a race.
void startRace(int raceID)
Starts a race.
void finishCurrentRace()
Finishes a race.
string lastCheckpointInstance
void unhideRace(int raceID)
This shows your race again, after it was hidden.
void addChpCoordinates(double[][] checkpoints_in, const string &in objName_checkpoint, const string &in objName_start, const string &in objName_finish, uint startNumber)
int truckNum
The number of the (last) used truck in the race.
void setCallback(string event, RACE_EVENT_CALLBACK @func)
Adds a callback funciton.
bool checkpointExists(int chpNum, int instance)
string lastRaceEventInstance
double[] bestTimeTillPoint
int state
In which state are we?
void destroy()
this function removes all checkpoints again
bool allowVehicleChanging
if false: if the user changes vehicle, the race will be aborted. (default=false)
int currentRace
the ID of the current race. (-1 is there's no race running at the moment)
bool showBestRace
if true: If a race is started or a new best race is set, the best race will be shown....