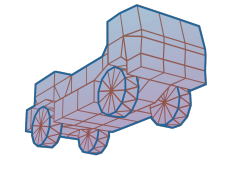 |
RigsofRods
Soft-body Physics Simulation
|
float getRotation()
Returns the angle in which the truck is heading.
VehicleAiClass getVehicleAI()
Retrieve the waypoint AI object.
bool getBrakeLightVisible()
Returns true if the brake light is enabled.
void setBlinkType(int blink)
Sets the blinking type.
vector3 isNodeWheelRim(int nodeNumber)
Is node marked as wheel rim? Note some wheel models use only tire nodes.
Binding of RoR::Actor; a softbody-physics gameplay object, can be anything from soda can to space shu...
string getSectionConfig()
Gets the name of the loaded section for a truck.
bool isLocked()
Returns true if a hook of this truck is locked.
int countCustomLights(int)
Counts flares using the given custom light group number (1-10).
void parkingbrakeToggle()
Toggles the parking brake.
float getTotalMass(bool withLocked)
Gets the total mass of the truck.
Pseudo-namespace; it doesn't exist in code or script runtime, only in this documentation.
int getInstanceId()
Gets the unique Actor Instance ID; The same value as provided by Game2Script::eventCallbackEx().
void antilockbrakeToggle()
Toggles the anti-lock brakes.
bool getCustomLightVisible(int number)
Returns true if the custom light with the number number is enabled.
void toggleCustomParticles()
Toggles the custom particles.
vector3 getGForces()
Gets the G-forces that this truck is currently experiencing.
void setCustomLightVisible(int number, bool visible)
Enables or disables the custom light.
FlareType
Binding of RoR::FlareType.
vector3 getNodePosition(int nodeNumber)
Returns the position of the node.
vector3 isNodeWheelTire(int nodeNumber)
Is node marked as wheel tire? Note some wheel models use only tire nodes.
TruckState getTruckState()
float getHeadingDirectionAngle()
Returns the angle in which the truck is heading.
void scaleTruck(float ratio)
Scales the truck.
float getWheelSpeed()
Gets the current wheel speed of the vehicle.
bool getBeaconMode()
Gets the mode of the beacon.
quaternion getOrientation() float getSpeed()
Orientation on all axes packed to single quaternion.
void tractioncontrolToggle()
Toggles the tracktion control.
string getTruckName()
Gets the designated name of the truck.
int getWheelNodeCount()
Gets the total amount of nodes of the wheels of the truck.
int countFlaresByType(FlareType)
Counts flares using the given type.
vector3 getPosition()
Get vehicle absolute position.
int getBlinkType()
Gets the blinking type.
void beaconsToggle()
Toggles the beacons.
void reset(bool keep_position)
Resets the truck.
int getNodeCount()
Gets the total amount of nodes of the truck.
void setMass(float m)
Sets the mass of the truck.
bool getReverseLightVisible()
Returns true if the reverse lights are enabled.
vector3 getVehiclePosition()
string getTruckFileName()
Gets the name of the truck definition file.
string getTruckFileResourceGroup()
Gets the name of the OGRE resource group where the truck definition file lives.
bool getCustomParticleMode()
Gets the custom particles mode.