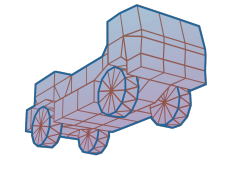 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
32 #include "utf8/checked.h"
33 #include "utf8/unchecked.h"
36 #include <OgreUTFString.h>
40 Ogre::String
sha1sum(
const char *key,
int len);
42 Ogre::String
HashData(
const char *key,
int len);
54 std::string::size_type pos = 0;
55 while ((pos = str.find(searchString, pos)) != std::string::npos)
62 Ogre::Real
Round(Ogre::Real value,
unsigned short ndigits = 0);
67 inline std::string&
TrimStr(std::string& s) { Ogre::StringUtil::trim(s);
return s; }
68 std::string
Sha1Hash(std::string
const & data);
70 std::string
JoinStrVec(Ogre::StringVector tokens,
const std::string& delim);
73 template <
class T,
class A,
class Predicate>
74 inline void EraseIf(std::vector<T, A>& c, Predicate pred)
76 c.erase(std::remove_if(c.begin(), c.end(), pred), c.end());
89 Ogre::Vector3
Convert(Ogre::Vector3 world_pos)
93 float screen_x = ((clip_space_pos.x / 2.f) + 0.5f) *
m_screen_size.x;
94 float screen_y = (1.f - ((clip_space_pos.y / 2.f) + 0.5f)) *
m_screen_size.y;
95 return Ogre::Vector3(screen_x, screen_y, view_space_pos.z);
104 bool IsDistanceWithin(Ogre::Vector3
const& a, Ogre::Vector3
const& b,
float max);
106 std::string
PrintMeshInfo(std::string
const& title, Ogre::MeshPtr mesh);
Ogre::String getVersionString(bool multiline=true)
Ogre::Matrix4 m_projection_matrix
std::string PrintMeshInfo(std::string const &title, Ogre::MeshPtr mesh)
std::string SanitizeUtf8String(std::string const &str_in)
Ogre::Real Round(Ogre::Real value, unsigned short ndigits=0)
Ogre::String HashData(const char *key, int len)
std::string & TrimStr(std::string &s)
Ogre::Vector3 Convert(Ogre::Vector3 world_pos)
void replaceString(std::string &str, std::string searchString, std::string replaceString)
std::time_t getTimeStamp()
Ogre::UTFString formatBytes(double bytes)
< Keeps data close for faster access.
Ogre::Vector2 m_screen_size
Central state/object manager and communications hub.
void CvarAddFileToList(CVar *cvar, const std::string &filename)
Quake-style console variable, defined in RoR.cfg or crated via Console UI and scripts.
std::string SanitizeUtf8CString(const char *start, const char *end=nullptr)
std::string JoinStrVec(Ogre::StringVector tokens, const std::string &delim)
Ogre::Matrix4 m_view_matrix
std::string Sha1Hash(std::string const &data)
void CvarRemoveFileFromList(CVar *cvar, const std::string &filename)
World2ScreenConverter(Ogre::Matrix4 view_mtx, Ogre::Matrix4 proj_mtx, Ogre::Vector2 screen_size)
void EraseIf(std::vector< T, A > &c, Predicate pred)
Ogre::UTFString tryConvertUTF(const char *buffer)
Ogre::String sha1sum(const char *key, int len)
bool IsDistanceWithin(Ogre::Vector3 const &a, Ogre::Vector3 const &b, float max)