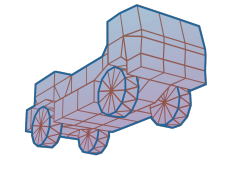 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
30 LocalStorage(
string filename,
const string&in sectionname =
"common",
const string&in resource_group_name =
"Cache");
40 string get(
string key);
50 void set(
string key,
const string value);
57 void setString(
string key,
const string value);
60 void set(
string key,
float value);
61 void setFloat(
string key,
float value);
64 void set(
string key,
const vector3 value);
65 void setVector3(
string key,
const vector3 value);
68 void set(
string key,
const radian value);
69 void setRadian(
string key,
const radian value);
72 void set(
string key,
const degree value);
73 void setDegree(
string key,
const degree value);
80 void set(
string key,
const bool value);
81 void setBool(
string key,
const bool value);
85 void set(
string key,
int);
86 void setInt(
string key,
int);
105 bool exists(
string key)
const;
111 void delete(
string key);
void save()
This saves the data to a file.
string getString(string key)
void setBool(string key, const bool value)
void setVector3(string key, const vector3 value)
Binding of RoR::LocalStorage; A class that allows your script to store data persistently.
void setFloat(string key, float value)
Pseudo-namespace; it doesn't exist in code or script runtime, only in this documentation.
bool exists(string key) const
Check if certain key exists.
void setRadian(string key, const radian value)
LocalStorage(string filename, const string &in sectionname="common", const string &in resource_group_name="Cache")
The constructor for the class.
vector3 getVector3(string key)
degree getDegree(string key)
void setQuaternion(string key, const quaternion value)
void setInt(string key, int)
void changeSection(const string section)
Changes the default section.
void setInteger(string key, int)
radian getRadian(string key)
string get(string key)
A getter for string data.
float getFloat(string key)
int getInteger(string key)
void setString(string key, const string value)
This sets a key.
quaternion getQuaternion(string key)
void set(string key, const string value)
void setDegree(string key, const degree value)
bool reload()
This reloads the data from the saved file, overwriting your changes.