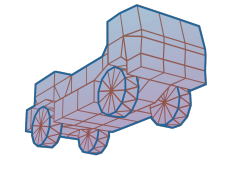 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
25 #include <condition_variable>
110 int logical_cores = std::thread::hardware_concurrency();
113 if (num_threads < 1 || num_threads > logical_cores)
115 num_threads = Ogre::Math::Clamp(logical_cores - 1, 1, 8);
119 RoR::LogFormat(
"[RoR|ThreadPool] Found %d logical CPU cores, creating %d worker threads",
120 logical_cores, num_threads);
137 auto thread_body = [
this]{
155 std::lock_guard<std::mutex> task_lock(current_task->m_task_mutex);
156 current_task->m_task_func();
157 current_task->m_is_finished =
true;
159 current_task->m_finish_cv.notify_all();
164 for (
int i = 0; i < num_threads; ++i) {
178 std::shared_ptr<Task>
RunTask(
const std::function<
void()> &task_func) {
181 auto task = std::shared_ptr<Task>(
new Task(task_func));
193 void Parallelize(
const std::vector<std::function<
void()>> &task_funcs)
195 if (task_funcs.empty())
return;
198 auto it = begin(task_funcs);
199 const auto first_task = it++;
200 std::vector<std::shared_ptr<Task>> handles;
201 for(; it != end(task_funcs); ++it)
203 handles.push_back(
RunTask(*it));
210 for(
const auto &h : handles) { h->join(); }
#define ROR_ASSERT(_EXPR)
std::vector< std::thread > m_threads
Collection of worker threads to run tasks.
std::queue< std::shared_ptr< Task > > m_taskqueue
Queue of submitted tasks pending for execution.
bool m_is_finished
Indicates whether the task execution has finished.
void LogFormat(const char *format,...)
Improved logging utility. Uses fixed 2Kb buffer.
Task(std::function< void()> task_func)
void join() const
Block the current thread and wait for the associated task to finish.
Task & operator=(Task &)=delete
Facilitates execution of (small) tasks on separate threads.
Central state/object manager and communications hub.
const std::function< void()> m_task_func
Callable object which implements the task to execute.
std::mutex m_taskqueue_mutex
Protects task queue from concurrent access.
std::shared_ptr< Task > RunTask(const std::function< void()> &task_func)
Submit new asynchronous task to thread pool and return Task handle to allow for synchronization.
/brief Handle for a task executed by ThreadPool
std::atomic_bool m_terminate
Indicates destruction of ThreadPool instance to worker threads.
ThreadPool(int num_threads)
Construct thread pool and launch worker threads.
std::condition_variable m_task_available_cv
Used to signal threads that a new task was submitted and is ready to run.
std::mutex m_task_mutex
Mutex which is locked while the task is running.
void Parallelize(const std::vector< std::function< void()>> &task_funcs)
Run collection of tasks in parallel and wait until all have finished.
std::condition_variable m_finish_cv
Used to signal the current thread when the task has finished.
static ThreadPool * DetectNumWorkersAndCreate()