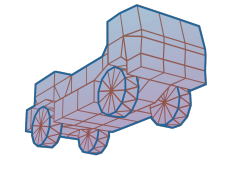 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
30 #include <angelscript.h>
31 #include <scriptdictionary/scriptdictionary.h>
53 void log(
const Ogre::String& msg);
67 int useOnlineAPI(
const Ogre::String& apiquery,
const AngelScript::CScriptDictionary& dict, Ogre::String& result);
96 bool deleteResource(
const std::string& filename,
const std::string& resource_group);
115 bool createTextResourceFromString(
const std::string& data,
const std::string& filename,
const std::string& resource_group,
bool overwrite=
false);
122 AngelScript::CScriptArray*
findResourceFileInfo(
const std::string& resource_group,
const std::string& pattern,
bool dirs =
false);
127 Ogre::Image
loadImageResource(
const std::string& filename,
const std::string& resource_group);
132 bool serializeMeshResource(
const std::string& filename,
const std::string& resource_group,
const Ogre::MeshPtr& mesh);
143 void flashMessage(Ogre::String& txt,
float time,
float charHeight);
148 void message(Ogre::String& txt, Ogre::String& icon);
160 void showMessageBox(Ogre::String& title, Ogre::String& text,
bool use_btn1, Ogre::String& btn1_text,
bool allow_close,
bool use_btn2, Ogre::String& btn2_text);
162 void showChooser(
const Ogre::String& type,
const Ogre::String&
instance,
const Ogre::String& box);
341 void spawnObject(
const Ogre::String& objectName,
const Ogre::String& instanceName,
const Ogre::Vector3& pos,
const Ogre::Vector3& rot,
const Ogre::String& eventhandler,
bool uniquifyMaterials);
349 void moveObjectVisuals(
const Ogre::String& instanceName,
const Ogre::Vector3& pos);
457 ActorPtr spawnTruckAI(Ogre::String& truckName, Ogre::Vector3& pos, Ogre::String& truckSectionConfig, std::string& truckSkin,
int x);
567 int setMaterialAmbient(
const Ogre::String& materialName,
float red,
float green,
float blue);
568 int setMaterialDiffuse(
const Ogre::String& materialName,
float red,
float green,
float blue,
float alpha);
569 int setMaterialSpecular(
const Ogre::String& materialName,
float red,
float green,
float blue,
float alpha);
570 int setMaterialEmissive(
const Ogre::String& materialName,
float red,
float green,
float blue);
571 int setMaterialTextureName(
const Ogre::String& materialName,
int techniqueNum,
int passNum,
int textureUnitNum,
const Ogre::String& textureName);
572 int setMaterialTextureRotate(
const Ogre::String& materialName,
int techniqueNum,
int passNum,
int textureUnitNum,
float rotation);
573 int setMaterialTextureScroll(
const Ogre::String& materialName,
int techniqueNum,
int passNum,
int textureUnitNum,
float sx,
float sy);
574 int setMaterialTextureScale(
const Ogre::String& materialName,
int techniqueNum,
int passNum,
int textureUnitNum,
float u,
float v);
600 std::string
CheckFileAccess(
const char* func_name,
const std::string& filename,
const std::string& resource_group);
601 int getTextureUnitState(Ogre::TextureUnitState** tu,
const Ogre::String materialName,
int techniqueNum,
int passNum,
int textureUnitNum);
bool HavePlayerAvatar(const char *func_name)
Helper; Check if local Character instance exists, log warning if not.
std::string getAIVehicleSkin(int x)
SoundScriptTemplatePtr getSoundScriptTemplate(const std::string &name)
AngelScript::CScriptArray * getAllSoundScriptTemplates()
void setCameraPosition(const Ogre::Vector3 &pos)
Sets the camera's position.
int sendGameCmd(const Ogre::String &message)
Multiplayer only: sends AngelScript snippet to all players.
void setAIRepeatTimes(int times)
int setMaterialTextureScale(const Ogre::String &materialName, int techniqueNum, int passNum, int textureUnitNum, float u, float v)
int getLoadedTerrain(Ogre::String &result)
gets the name of current terrain.
int getCurrentTruckNumber()
returns the current truck number.
int setMaterialTextureRotate(const Ogre::String &materialName, int techniqueNum, int passNum, int textureUnitNum, float rotation)
int setMaterialTextureScroll(const Ogre::String &materialName, int techniqueNum, int passNum, int textureUnitNum, float sx, float sy)
SoundScriptInstancePtr createSoundScriptInstance(const std::string &template_name, int actor_instance_id)
void setCameraOrientation(const Ogre::Quaternion &q)
Sets the camera's orientation.
float getTime()
returns the time in seconds since the game was started
void setWaterHeight(float value)
sets the base water height
void setPersonRotation(const Ogre::Radian &rot)
sets the character rotation
int setMaterialDiffuse(const Ogre::String &materialName, float red, float green, float blue, float alpha)
bool pushMessage(MsgType type, AngelScript::CScriptDictionary *dict)
Pushes a message to internal message queue.
void setCameraPitch(float angle)
Pitches the camera up/down anticlockwise around it's local z axis.
float getWaterHeight()
returns the current base water level (without waves)
int getAIVehicleDistance()
void updateDirectionArrow(Ogre::String &text, Ogre::Vector3 &vec)
set direction arrow
AngelScript::CScriptArray * getWaypointsSpeed()
void flashMessage(Ogre::String &txt, float time, float charHeight)
DEPRECATED: use message() shows a message to the user.
void setAIVehicleName(int x, std::string name)
int getNumTrucksByFlag(int flag)
void setAIVehicleSkin(int x, std::string skin)
bool getCaelumAvailable()
void activateAllVehicles()
int setMaterialTextureName(const Ogre::String &materialName, int techniqueNum, int passNum, int textureUnitNum, const Ogre::String &textureName)
VehicleAIPtr getCurrentTruckAI()
bool getMousePositionOnTerrain(Ogre::Vector3 &out_pos)
Calculates mouse cursor position on terrain.
Ogre::Vector3 getPersonPosition()
void loadTerrain(const Ogre::String &terrain)
ActorPtr getTruckByNum(int num)
returns a truck by index, get max index by calling getNumTrucks
Ogre::SceneManager * getSceneManager()
bool HaveSimTerrain(const char *func_name)
Helper; Check if SimController instance exists, log warning if not.
float getGroundHeight(Ogre::Vector3 &v)
Gets terrain height at given coordinates.
Ogre::String getAIVehicleName(int x)
void openUrlInDefaultBrowser(const std::string &url)
Opens URL (must start with 'http://' or 'https://') in system's default web browser.
AngelScript::CScriptArray * getWaypoints(int x)
void setCameraDirection(const Ogre::Vector3 &vec)
Sets the camera's direction vector.
void setAIVehiclePositionScheme(int scheme)
Ogre::Image loadImageResource(const std::string &filename, const std::string &resource_group)
Loads an image in any format recognized by OGRE.
float getGravity()
returns the currently set upo gravity
BitMask_t getRegisteredEventsMask(ScriptUnitId_t nid)
Gets event mask for a specific running script.
void removeVehicle(const Ogre::String &instance, const Ogre::String &box)
void showChooser(const Ogre::String &type, const Ogre::String &instance, const Ogre::String &box)
void spawnObject(const Ogre::String &objectName, const Ogre::String &instanceName, const Ogre::Vector3 &pos, const Ogre::Vector3 &rot, const Ogre::String &eventhandler, bool uniquifyMaterials)
This spawns a static terrain object (.ODEF file)
ActorPtr getCurrentTruck()
returns the current selected truck, 0 if in person mode
bool HaveMainCamera(const char *func_name)
Helper; Check if main camera exists, log warning if not.
void boostCurrentTruck(float factor)
Ogre::String getCaelumTime()
gets the time of the day in seconds
Ogre::Quaternion getCameraOrientation()
Gets the camera's orientation.
int setMaterialSpecular(const Ogre::String &materialName, float red, float green, float blue, float alpha)
void message(Ogre::String &txt, Ogre::String &icon)
shows a message to the user over the console system
Ogre::Vector3 getCameraPosition()
Retrieves the camera's position.
Ogre::String getAIVehicleSectionConfig(int x)
AngelScript::CScriptDictionary * getScriptDetails(ScriptUnitId_t nid)
Returns all info about running script; obtain the NID from getRunningScripts(), global var thisScript...
void setTimeDiff(float diff)
AngelScript::CScriptArray * findResourceFileInfo(const std::string &resource_group, const std::string &pattern, bool dirs=false)
Proxy to Ogre::ResourceGroupManager::findResourceFileInfo(), see https://ogrecave....
void setGravity(float value)
sets the gravity terrain wide.
void setBestLapTime(float time)
int setMaterialAmbient(const Ogre::String &materialName, float red, float green, float blue)
int ScriptUnitId_t
Unique sequentially generated ID of a loaded and running scriptin session. Use ScriptEngine::getScrip...
void showMessageBox(Ogre::String &title, Ogre::String &text, bool use_btn1, Ogre::String &btn1_text, bool allow_close, bool use_btn2, Ogre::String &btn2_text)
std::string CheckFileAccess(const char *func_name, const std::string &filename, const std::string &resource_group)
Extract filename and extension from the input, because OGRE allows absolute paths in resource system.
int useOnlineAPI(const Ogre::String &apiquery, const AngelScript::CScriptDictionary &dict, Ogre::String &result)
int FreeForceID_t
Unique sequentially generated ID of FreeForce; use ActorManager::GetFreeForceNextId().
void setPersonPosition(const Ogre::Vector3 &vec)
sets the character position
void destroyObject(const Ogre::String &instanceName)
This destroys an object.
void setCaelumTime(float value)
sets the time of the day in seconds
MsgType
Global gameplay message loop, see struct Message in GameContext.h.
void registerForEvent(int eventValue)
registers for a new event to be received by the scripting system
Central state/object manager and communications hub.
void setAIVehicleDistance(int dist)
void log(const Ogre::String &msg)
writes a message to the games log (RoR.log)
Ogre::Vector3 getCameraDirection()
Gets the camera's direction.
void setCameraYaw(float angle)
Rotates the camera anticlockwise around it's local y axis.
ActorPtr spawnTruckAI(Ogre::String &truckName, Ogre::Vector3 &pos, Ogre::String &truckSectionConfig, std::string &truckSkin, int x)
void fetchUrlAsStringAsync(const std::string &url, const std::string &display_filename)
Invokes a background thread to fetch data using CURL; when finished, sends MSG_APP_SCRIPT_THREAD_STAT...
bool serializeMeshResource(const std::string &filename, const std::string &resource_group, const Ogre::MeshPtr &mesh)
Uses Ogre::MeshSerializer to save binary .mesh file (latest format, native endianness).
bool createTextResourceFromString(const std::string &data, const std::string &filename, const std::string &resource_group, bool overwrite=false)
Saves a string as a text file resource.
AngelScript::CScriptArray * getAllTrucks()
returns an array of all currently existing actors.
Ogre::Radian getPersonRotation()
gets the character rotation
Ogre::Vector2 getDisplaySize()
Gets screen size in pixels.
int getChatFontSize()
OBSOLETE, returns 0;.
int setMaterialEmissive(const Ogre::String &materialName, float red, float green, float blue)
int scriptFunctionExists(const Ogre::String &arg)
Checks if a global function exists in the script (Wrapper for ScriptEngine::functionExists)
AngelScript::CScriptArray * getAllSoundScriptInstances()
void setAIVehicleSectionConfig(int x, std::string config)
void hideDirectionArrow()
int deleteScriptFunction(const Ogre::String &arg)
Deletes a global function from the script (Wrapper for ScriptEngine::deleteFunction)
Proxy class that can be called by script functions.
or anywhere else will not be considered a but parsed as regular data ! Each line is treated as values separated by separators Possible i e animators Multiline description Single instance
VehicleAIPtr getTruckAIByNum(int num)
AngelScript::CScriptArray * getRunningScripts()
Returns array<int> with active ScriptUnitIDs; check agains global var thisScript or use getScriptDeta...
void moveObjectVisuals(const Ogre::String &instanceName, const Ogre::Vector3 &pos)
This moves an object to a new position.
void addWaypoint(const Ogre::Vector3 &pos)
FreeForceID_t getFreeForceNextId()
Returns an unused (not reused) ID to use with MSG_SIM_ADD_FREEFORCE_REQUESTED; see game....
int getAIVehiclePositionScheme()
void movePerson(const Ogre::Vector3 &vec)
moves the person relative
void setCameraRoll(float angle)
Rolls the camera anticlockwise, around its local z axis.
bool checkResourceExists(const std::string &filename, const std::string &resource_group)
Checks if the resource file exists in the given group.
int getTextureUnitState(Ogre::TextureUnitState **tu, const Ogre::String materialName, int techniqueNum, int passNum, int textureUnitNum)
void setAIVehicleSpeed(int speed)
void setTrucksForcedAwake(bool forceActive)
SoundPtr createSoundFromResource(const std::string &filename, const std::string &resource_group_name)
int addScriptFunction(const Ogre::String &arg)
Adds a global function to the script (Wrapper for ScriptEngine::addFunction)
std::string loadTextResourceAsString(const std::string &filename, const std::string &resource_group)
Loads a text file resource as string.
void setRegisteredEventsMask(ScriptUnitId_t nid, BitMask_t eventMask)
Overwrites event mask for a specific running script.
bool getScreenPosFromWorldPos(Ogre::Vector3 const &world_pos, Ogre::Vector2 &out_screen_pos)
void repairVehicle(const Ogre::String &instance, const Ogre::String &box, bool keepPosition)
bool deleteResource(const std::string &filename, const std::string &resource_group)
Deletes a resource from the given group.
int deleteScriptVariable(const Ogre::String &arg)
Deletes a global variable from the script (Wrapper for ScriptEngine::deleteVariable)
void setChatFontSize(int size)
OBSOLETE, does nothing.
ActorPtr spawnTruck(Ogre::String &truckName, Ogre::Vector3 &pos, Ogre::Vector3 &rot)
void unRegisterEvent(int eventValue)
unregisters from receiving event.
int addScriptVariable(const Ogre::String &arg)
Adds a global variable to the script (Wrapper for ScriptEngine::addVariable)
void cameraLookAt(const Ogre::Vector3 &targetPoint)
Points the camera at a location in worldspace.
void setAIVehicleCount(int count)
Ogre::Vector2 getMouseScreenPosition()
Gets mouse position in pixels.
void logFormat(const char *fmt,...)
writes a message to the games log (RoR.log)
float rangeRandom(float from, float to)