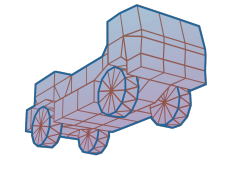 |
RigsofRods
2023.09
Soft-body Physics Simulation
|
Go to the documentation of this file.
23 #ifdef USE_ANGELSCRIPT
36 #include "scriptdictionary/scriptdictionary.h"
79 item.GetValue(&point, item.GetTypeId());
80 std::string key(item.GetKey());
87 Ogre::Vector3 translation = Ogre::Vector3::ZERO;
93 translation.x += offset * cos(
beam->
getRotation() - Ogre::Math::HALF_PI);
94 translation.z += offset * sin(
beam->
getRotation() - Ogre::Math::HALF_PI);
107 translation -= offset * dir.normalisedCopy();
112 float angle = Ogre::Vector3::UNIT_Z.angleBetween(dir.normalisedCopy()).valueRadians();
116 angle = -Ogre::Vector3::UNIT_Z.angleBetween(dir.normalisedCopy()).valueRadians();
119 translation.x -= offset * cos(angle);
120 translation.z -= offset * sin(angle);
230 mAgentPosition.y = 0;
252 angle_rad = dir1.angleBetween(dir2.normalisedCopy()).valueRadians();
253 angle_deg = dir1.angleBetween(dir2.normalisedCopy()).valueDegrees();
282 TargetPosition.y = 0;
283 Quaternion TargetOrientation = Quaternion::ZERO;
285 Quaternion mAgentOrientation = Quaternion(Radian(
beam->
getRotation()), Vector3::NEGATIVE_UNIT_Y);
286 mAgentOrientation.normalise();
288 Vector3 mVectorToTarget = TargetPosition - mAgentPosition;
289 mAgentPosition.normalise();
291 Vector3 mAgentHeading = mAgentOrientation * mAgentPosition;
292 Vector3 mTargetHeading = TargetOrientation * TargetPosition;
293 mAgentHeading.normalise();
294 mTargetHeading.normalise();
297 Vector3 mSteeringForce = mAgentOrientation.Inverse() * mVectorToTarget;
298 mSteeringForce.normalise();
300 float mYaw = mSteeringForce.x;
301 float mPitch = mSteeringForce.z;
356 if (abs(mYaw) < 0.5f)
363 else if (kmh_wheel_speed >
maxspeed + 1)
381 else if (kmh_wheel_speed >
maxspeed + 1)
404 float t = ((angle_deg - 10) / (180 - 10))*1.4f;
434 if (
beam->
getPosition().distance(actor->getPosition()) < kmh_wheel_speed)
442 for (
int k = 0; k < actor->ar_num_nodes; k++)
600 if (abs(mYaw) < 0.5f)
651 #endif // USE_ANGELSCRIPT
Game state manager and message-queue provider.
void addEvent(std::string const &id, int ev)
Adds a event.
float acc_power
The engine power.
NodeNum_t ar_main_camera_node_pos
Sim attr; ar_camera_node_pos[0] >= 0 ? ar_camera_node_pos[0] : 0.
float getWheelSpeed() const
void addWaypoint(std::string const &id, Ogre::Vector3 const &point)
Adds one waypoint.
Ogre::Vector3 current_waypoint
The coordinates of the waypoint that the AI is driving to.
Ogre::Vector3 next_waypoint
The coordinates of the previous waypoint.
GUIManager * GetGuiManager()
int current_waypoint_id
The coordinates of the next waypoint.
Ogre::Vector3 GetCameraDir()
Character * GetPlayerCharacter()
Ogre::Real ar_brake
Physics state; braking intensity.
ActorPtr GetActorCoupling()
void putMessage(MessageArea area, MessageType type, std::string const &msg, std::string icon="")
int ar_aerial_flap
Sim state; state of aircraft flaps (values: 0-5)
void setRudder(float val)
@ NOT_DRIVEABLE
not drivable at all
Ogre::Vector3 prev_waypoint
virtual void flipStart()=0
bool is_enabled
True if the AI is driving.
AeroEngine * ar_aeroengines[MAX_AEROENGINES]
bool isActive()
Returns the status of the AI.
Screwprop * ar_screwprops[MAX_SCREWPROPS]
std::map< int, std::string > waypoint_names
Map with all waypoint names.
void setValueAtWaypoint(std::string const &id, int value_id, float value)
Sets a value at a waypoint.
Ogre::Vector3 getTranslation(int offset, unsigned int wp)
Gets offset translation based on vehicle rotation and waypoints.
Ogre::Vector3 getPosition()
void autoSetAcc(float val)
void setThrottle(float val)
GameContext * GetGameContext()
Ogre::Vector3 getPosition()
int free_waypoints
The amount of waypoints.
Ogre::Vector3 getDirection()
average actor velocity, calculated using the actor positions of the last two frames
void updateWaypoint()
Updates the AI waypoint.
GUI::TopMenubar TopMenubar
Ogre::Vector3 GetCameraRoll()
float wait_time
(seconds) The amount of time the AI has to wait.
float maxspeed
(KM/H) The max speed the AI is allowed to drive.
float ar_elevator
Sim state; aerial controller.
ActorPtr beam
The verhicle the AI is driving.
std::map< int, int > waypoint_events
Map with all waypoint events.
virtual bool getIgnition()=0
float ar_hydro_dir_command
Ogre::Vector3 getNodePosition(int nodeNumber)
Returns world position of node.
virtual void setThrottle(float val)=0
void addWaypoints(AngelScript::CScriptDictionary &d)
Adds a dictionary with waypoints.
@ CONSOLE_MSGTYPE_SCRIPT
Messages sent from scripts.
virtual ~VehicleAI() override
float ar_aileron
Sim state; aerial controller.
const ActorPtr & GetPlayerActor()
void startEngine()
Quick engine start. Plays sounds.
std::map< Ogre::String, float > waypoint_speed
Map with all waypoint speeds.
std::map< std::string, int > waypoint_ids
Map with all waypoint IDs.
void update(float dt, int doUpdate)
Updates the AI.
void setActive(bool value)
Activates/Deactivates the AI.
std::map< int, Ogre::Vector3 > waypoints
Map with all waypoints.
std::map< int, float > waypoint_power
Map with all waypoint engine power.