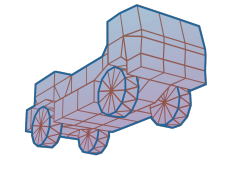 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
30 #ifdef USE_ANGELSCRIPT
35 #include "scriptdictionary/scriptdictionary.h"
87 void addWaypoint(std::string
const&
id, Ogre::Vector3
const& point);
103 void addEvent(std::string
const&
id,
int ev);
129 void update(
float dt,
int doUpdate);
163 #endif // USE_ANGELSCRIPT
void addEvent(std::string const &id, int ev)
Adds a event.
float acc_power
The engine power.
void addWaypoint(std::string const &id, Ogre::Vector3 const &point)
Adds one waypoint.
Ogre::Vector3 current_waypoint
The coordinates of the waypoint that the AI is driving to.
Ogre::Vector3 next_waypoint
The coordinates of the previous waypoint.
Ai_values
Enum with AI values that can be set.
int current_waypoint_id
The coordinates of the next waypoint.
Ogre::Vector3 prev_waypoint
bool is_enabled
True if the AI is driving.
bool isActive()
Returns the status of the AI.
std::map< int, std::string > waypoint_names
Map with all waypoint names.
void setValueAtWaypoint(std::string const &id, int value_id, float value)
Sets a value at a waypoint.
Ogre::Vector3 getTranslation(int offset, unsigned int wp)
Gets offset translation based on vehicle rotation and waypoints.
std::map< int, float > waypoint_wait_time
Map with all waypoint wait times.
Central state/object manager and communications hub.
int free_waypoints
The amount of waypoints.
Ai_events
Enum with AI events.
void updateWaypoint()
Updates the AI waypoint.
float wait_time
(seconds) The amount of time the AI has to wait.
float maxspeed
(KM/H) The max speed the AI is allowed to drive.
ActorPtr beam
The verhicle the AI is driving.
std::map< int, int > waypoint_events
Map with all waypoint events.
void addWaypoints(AngelScript::CScriptDictionary &d)
Adds a dictionary with waypoints.
Self reference-counting objects, as requred by AngelScript garbage collector.
virtual ~VehicleAI() override
std::map< Ogre::String, float > waypoint_speed
Map with all waypoint speeds.
std::map< std::string, int > waypoint_ids
Map with all waypoint IDs.
void update(float dt, int doUpdate)
Updates the AI.
void setActive(bool value)
Activates/Deactivates the AI.
std::map< int, Ogre::Vector3 > waypoints
Map with all waypoints.
std::map< int, float > waypoint_power
Map with all waypoint engine power.