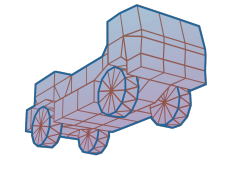 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
51 Ogre::Quaternion
rotation = Ogre::Quaternion::IDENTITY;
71 void setName(std::string
const& new_name) {
name = new_name; }
74 std::vector<ProceduralPointPtr>
points;
void addObject(ProceduralObjectPtr po)
Generates road mesh and adds to internal list.
void removeObject(ProceduralObjectPtr po)
Clears road mesh and removes from internal list.
std::vector< ProceduralPointPtr > points
std::vector< ProceduralObjectPtr > pObjects
void deletePoint(int pos)
Ogre::Quaternion rotation
virtual ~ProceduralManager() override
ProceduralManager(Ogre::SceneNode *groupingSceneNode)
void updateObject(ProceduralObjectPtr po)
Rebuilds the road mesh.
virtual ~ProceduralObject() override
void setName(std::string const &new_name)
void addPoint(ProceduralPointPtr p)
Ogre::SceneNode * pGroupingSceneNode
virtual ~ProceduralPoint() override
ProceduralObjectPtr getObject(int pos)
Central state/object manager and communications hub.
void insertPoint(int pos, ProceduralPointPtr p)
ProceduralPointPtr getPoint(int pos)
void deleteObject(ProceduralObjectPtr po)
Deletes the road mesh.
ProceduralRoadPtr getRoad()
Self reference-counting objects, as requred by AngelScript garbage collector.
ProceduralPoint(const ProceduralPoint &orig)