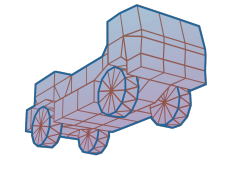 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
100 void pushNetworkState(
float engine_rpm,
float acc,
float clutch,
int gear,
bool running,
bool contact,
char auto_mode,
char auto_select = -1);
float getPostShiftClock()
float getClutchTime() const
Time (in seconds) the clutch takes to apply ('engoption' attr #5)
float getAccToHoldRPM()
estimate required throttle input to hold the current rpm
void startEngine()
Quick engine start. Plays sounds.
void autoSetAcc(float val)
void offStart()
Quick start of vehicle engine.
void setAutoMode(SimGearboxMode mode)
void pushNetworkState(float engine_rpm, float acc, float clutch, int gear, bool running, bool contact, char auto_mode, char auto_select=-1)
void shiftTo(int val)
Changes gear to given value. Plays sounds.
void setWheelSpin(float rpm)
float getWheelSpin()
Current wheel RPM.
float getIdleRPM() const
('engoption' attr #8)
float getDiffRatio() const
Global gear ratio ('engine' attr #4)
float getGearRatio(int pos)
-1=R, 0=N, 1... ('engine' attrs #5[R],#6[N],#7[1]...)
Pseudo-namespace; it doesn't exist in code or script runtime, only in this documentation.
void toggleContact()
Ignition.
float getAutoShiftBehavior()
float getClutchForce() const
('engoption' attr #3)
void setClutch(float clutch)
float getShiftTime() const
Time (in seconds) that it takes to shift ('engoption' attr #4)
float getEngineTorque() const
Torque in N/m ('engine' attr #3)
void autoShiftSet(autoswitch mode)
bool hasContact()
Ignition.
float getMinIdleMixture() const
Minimum throttle to maintain the idle RPM ('engoption' attr #10)
float getPostShiftTime() const
Time (in seconds) until full torque is transferred ('engoption' attr #6)
float getShiftDownRPM() const
Shift down RPM ('engine' attr #1)
autoswitch getAutoShift()
void shift(int val)
Changes gear by a relative offset. Plays sounds.
float getEngineInertia() const
('engoption' attr #1)
float getStallRMP() const
('engoption' attr #7)
void setTCaseRatio(float ratio)
Set current transfer case gear (reduction) ratio.
void setManualClutch(float val)
SimGearboxMode getAutoMode()
char getEngineType() const
't' = truck (default), 'c' = car, 'e' = electric car ('engoption' attr #2)
void setGear(int v)
low level gear changing (bypasses shifting logic)
void setHydroPump(float work)
float getShiftUpRPM() const
Shift up RPM ('engine' attr #2)
int getKickdownDelayCounter()
Binding of RoR::EngineSim; A land vehicle engine + transmission.
int getUpshiftDelayCounter()
void stopEngine()
stall engine
int getNumGearsRanges() const
float getMaxIdleMixture() const
Maximum throttle to maintain the idle RPM ('engoption' attr #9)
float getBrakingTorque() const
void setGearRange(int v)
low level gear changing (bypasses shifting logic)