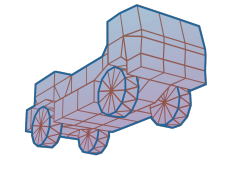 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
28 #include <OgreVector3.h>
29 #include <OgreString.h>
32 #include <OpenAL/al.h>
33 #include <OpenAL/alc.h>
58 void setCamera(Ogre::Vector3 position, Ogre::Vector3 direction, Ogre::Vector3 up, Ogre::Vector3 velocity);
75 void recomputeSource(
int source_index,
int reason,
float vfl, Ogre::Vector3 *vvec);
78 void assign(
int source_index,
int hardware_index);
79 void retire(
int source_index);
81 bool loadWAVFile(Ogre::String filename, ALuint buffer, Ogre::String resource_group_name =
"");
int audio_buffers_in_use_count
static const unsigned int MAX_HARDWARE_SOURCES
bool loadWAVFile(Ogre::String filename, ALuint buffer, Ogre::String resource_group_name="")
void assign(int source_index, int hardware_index)
void setMasterVolume(float v)
void recomputeSource(int source_index, int reason, float vfl, Ogre::Vector3 *vvec)
ALuint getHardwareSource(int hardware_index)
ALuint hardware_sources[MAX_HARDWARE_SOURCES]
static const float REFERENCE_DISTANCE
static const unsigned int MAX_AUDIO_BUFFERS
void retire(int source_index)
SoundPtr createSound(Ogre::String filename, Ogre::String resource_group_name="")
SoundPtr audio_sources[MAX_AUDIO_BUFFERS]
int getNumHardwareSources()
int hardware_sources_map[MAX_HARDWARE_SOURCES]
Central state/object manager and communications hub.
int hardware_sources_in_use_count
void recomputeAllSources()
ALCcontext * sound_context
Ogre::Vector3 camera_position
static const float MAX_DISTANCE
void setCamera(Ogre::Vector3 position, Ogre::Vector3 direction, Ogre::Vector3 up, Ogre::Vector3 velocity)
ALuint audio_buffers[MAX_AUDIO_BUFFERS]
Ogre::String audio_buffer_file_name[MAX_AUDIO_BUFFERS]
std::pair< int, float > audio_sources_most_audible[MAX_AUDIO_BUFFERS]
static const float ROLLOFF_FACTOR