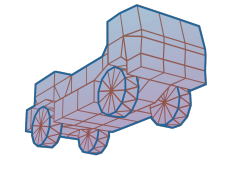 |
RigsofRods
Soft-body Physics Simulation
|
virtual ~Sound() override
void setPosition(Ogre::Vector3 pos)
void setPitch(float pitch)
int getCurrentHardwareIndex()
Central state/object manager and communications hub.
void setVelocity(Ogre::Vector3 vel)
void computeAudibility(Ogre::Vector3 pos)
Ogre::Vector3 getPosition()
Ogre::Vector3 getVelocity()
Self reference-counting objects, as requred by AngelScript garbage collector.
Sound(ALuint buffer, SoundManager *soundManager, int sourceIndex)
SoundManager * sound_manager