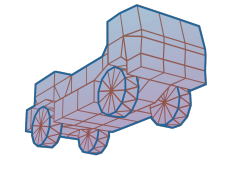 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
28 #include <angelscript.h>
41 LocalStorage(std::string filename,
const std::string& section_name,
const std::string& rg_name );
47 std::string
get(std::string key);
48 void set(std::string key,
const std::string& value);
50 int getInt(std::string key);
51 void set(std::string key,
const int value);
54 void set(std::string key,
const float value);
57 void set(std::string key,
const bool value);
60 void set(std::string key,
const Ogre::Vector3& value);
63 void set(std::string key,
const Ogre::Quaternion& value);
66 void set(std::string key,
const Ogre::Radian& value);
69 void set(std::string key,
const Ogre::Degree& value);
79 bool exists(std::string key);
85 void parseKey(std::string& inout_key, std::string& out_section);
bool saved
Inverted 'dirty flag'.
SettingsBySection getSettings()
Ogre::Vector3 getVector3(std::string key)
int getInt(std::string key)
Ogre::Radian getRadian(std::string key)
float getFloat(std::string key)
Used by AngelScript local storage.
A class that allows scripts to store data persistently.
std::string m_resource_group
Central state/object manager and communications hub.
std::string get(std::string key)
Ogre::Degree getDegree(std::string key)
void set(std::string key, const std::string &value)
void eraseKey(std::string key)
void parseKey(std::string &inout_key, std::string &out_section)
Ogre::Quaternion getQuaternion(std::string key)
void changeSection(const std::string §ion)
bool exists(std::string key)
virtual ~LocalStorage() override
Self reference-counting objects, as requred by AngelScript garbage collector.
bool getBool(std::string key)
std::string getFilename()
void copyFrom(LocalStoragePtr other)
LocalStorage(std::string filename, const std::string §ion_name, const std::string &rg_name)