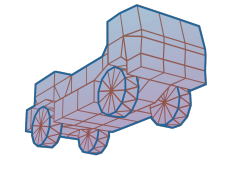 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
36 std::string allowedChars =
"abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789_-";
37 for (std::string::iterator it = fileName_in.begin() ; it < fileName_in.end() ; ++it){
38 if ( allowedChars.find(*it) == std::string::npos )
42 sectionName = sectionName_in.substr(0, sectionName_in.find(
".", 0));
62 SettingsBySection::iterator secIt;
63 SettingsBySection osettings = other->getSettings();
64 for (secIt = osettings.begin(); secIt!=osettings.end(); secIt++)
66 SettingsMultiMap::iterator setIt;
67 for (setIt = secIt->second->begin(); setIt!=secIt->second->end(); setIt++)
69 setSetting(setIt->first, setIt->second, secIt->first);
76 sectionName = section.substr(0, section.find(
".", 0));
212 catch (std::exception& e)
215 "Error saving file '%s' (resource group '%s'), message: '%s'",
226 catch (std::exception& e)
229 "Error reading file '%s' (resource group '%s'), message: '%s'",
241 if (mSettingsPtr.find(sec) != mSettingsPtr.end() && mSettingsPtr[sec]->find(key) != mSettingsPtr[sec]->end())
242 if (mSettingsPtr[sec]->erase(key) > 0)
253 size_t dot = key.find(
".", 0);
254 if ( dot != std::string::npos )
256 section = key.substr(0, dot);
258 if ( !section.length() )
Ogre::Real getSettingReal(Ogre::String key, Ogre::String section=Ogre::BLANKSTRING)
int getSettingInt(Ogre::String key, Ogre::String section=Ogre::BLANKSTRING)
bool saved
Inverted 'dirty flag'.
bool getSettingBool(Ogre::String key, Ogre::String section=Ogre::BLANKSTRING)
Ogre::Vector3 getVector3(std::string key)
bool saveImprovedCfg(std::string const &filename, std::string const &resource_group_name)
int getInt(std::string key)
Ogre::Radian getRadian(std::string key)
Ogre::Radian getSettingRadian(Ogre::String key, Ogre::String section=Ogre::BLANKSTRING)
void LogFormat(const char *format,...)
Improved logging utility. Uses fixed 2Kb buffer.
void loadImprovedCfg(std::string const &filename, std::string const &resource_group_name)
Ogre::String getString(Ogre::String const &key, Ogre::String const §ion, Ogre::String const &defaultValue="")
float getFloat(std::string key)
void setSetting(Ogre::String key, Ogre::String value, Ogre::String section=Ogre::BLANKSTRING)
std::string m_resource_group
Central state/object manager and communications hub.
std::string get(std::string key)
Ogre::Degree getDegree(std::string key)
void set(std::string key, const std::string &value)
void eraseKey(std::string key)
void parseKey(std::string &inout_key, std::string &out_section)
Ogre::Quaternion getQuaternion(std::string key)
Ogre::Vector3 getSettingVector3(Ogre::String key, Ogre::String section=Ogre::BLANKSTRING)
bool hasSetting(Ogre::String key, Ogre::String section="")
Ogre::Quaternion getSettingQuaternion(Ogre::String key, Ogre::String section=Ogre::BLANKSTRING)
void changeSection(const std::string §ion)
bool exists(std::string key)
virtual ~LocalStorage() override
bool getBool(std::string key)
void copyFrom(LocalStoragePtr other)
LocalStorage(std::string filename, const std::string §ion_name, const std::string &rg_name)