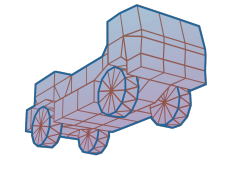 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
33 #include <OgreResourceManager.h>
47 Ogre::Vector3
tnt_pos = Ogre::Vector3::ZERO;
151 static std::vector<TuneupDefPtr>
ParseTuneups(Ogre::DataStreamPtr& stream);
bool isFlexbodyForceRemoved(FlexbodyID_t flexbodyid)
std::set< FlexbodyID_t > force_remove_flexbodies
UI overrides.
std::string twt_origin
Addonpart filename.
static bool isAddonPartUsed(TuneupDefPtr &tuneup_entry, const std::string &filename)
static std::vector< TuneupDefPtr > ParseTuneups(Ogre::DataStreamPtr &stream)
NodeNum_t tnt_nodenum
Arg#1, required.
static bool isWheelTweaked(TuneupDefPtr &tuneup_entry, WheelID_t wheel_id, TuneupWheelTweak *&out_tweak)
float twt_tire_radius
Arg#5, optional.
std::set< PropID_t > force_remove_props
UI overrides.
std::set< PropID_t > unwanted_props
'addonpart_unwanted_prop' directives.
< Data of 'addonpart_tweak_prop <prop ID> <offsetX> <offsetY> <offsetZ> <rotX> <rotY> <rotZ> <media1>...
float twt_rim_radius
Arg#6, optional, only applies to some wheel types.
Ogre::Vector3 tft_rotation
static bool isPropTweaked(TuneupDefPtr &tuneup_entry, PropID_t flexbody_id, TuneupPropTweak *&out_tweak)
std::string tft_origin
Addonpart filename.
static bool isPropAnyhowRemoved(TuneupDefPtr &tuneup_def, PropID_t prop_id)
std::map< PropID_t, TuneupPropTweak > prop_tweaks
Mesh name(s), offset and rotation overrides via 'addonpart_tweak_prop'.
static const NodeNum_t NODENUM_INVALID
static const PropID_t PROPID_INVALID
std::set< FlexbodyID_t > unwanted_flexbodies
'addonpart_unwanted_flexbody' directives.
static void ExportTuneup(Ogre::DataStreamPtr &stream, TuneupDefPtr &tuneup)
std::set< NodeNum_t > protected_nodes
Nodes that cannot be altered via 'addonpart_tweak_node'.
std::set< std::string > use_addonparts
Addonpart filenames.
bool isPropUnwanted(PropID_t propid)
static Ogre::Vector3 getTweakedPropOffset(TuneupDefPtr &tuneup_entry, PropID_t prop_id, Ogre::Vector3 orig_val)
static Ogre::Vector3 getTweakedPropRotation(TuneupDefPtr &tuneup_entry, PropID_t prop_id, Ogre::Vector3 orig_val)
bool isFlexbodyProtected(FlexbodyID_t flexbodyid)
Ogre::Vector3 tpt_rotation
std::set< PropID_t > protected_props
Props which cannot be altered via 'addonpart_tweak_prop' or 'addonpart_remove_prop' directive.
WheelID_t twt_wheel_id
Arg#1, required.
static bool isNodeTweaked(TuneupDefPtr &tuneup_entry, NodeNum_t nodenum, TuneupNodeTweak *&out_tweak)
static std::string getTweakedFlexbodyMediaRG(TuneupDefPtr &tuneup_def, FlexbodyID_t flexbody_id, int media_idx, const std::string &orig_val)
FlexbodyID_t tft_flexbody_id
std::map< NodeNum_t, TuneupNodeTweak > node_tweaks
Node position overrides via 'addonpart_tweak_node'.
static const FlexbodyID_t FLEXBODYID_INVALID
uint16_t NodeNum_t
Node position within Actor::ar_nodes; use RoR::NODENUM_INVALID as empty value.
static bool isFlexbodyAnyhowRemoved(TuneupDefPtr &tuneup_def, FlexbodyID_t flexbody_id)
bool isWheelProtected(int wheelid) const
< Data of 'addonpart_tweak_node <nodenum> <posX> <posY> <posZ>'
A database of user-installed content alias 'mods' (vehicles, terrains...)
static const WheelID_t WHEELID_INVALID
std::set< WheelID_t > protected_wheels
Wheels that cannot be altered via 'addonpart_tweak_wheel'.
WheelSide twt_side
Arg#4, optional, default LEFT (Only applicable to mesh/flexbody wheels)
static std::string getTweakedPropMedia(TuneupDefPtr &tuneup_entry, PropID_t prop_id, int media_idx, const std::string &orig_val)
std::map< FlexbodyID_t, TuneupFlexbodyTweak > flexbody_tweaks
Mesh name, offset and rotation overrides via 'addonpart_tweak_flexbody'.
int WheelID_t
Index to Actor::ar_wheels, use RoR::WHEELID_INVALID as empty value.
Ogre::Vector3 tnt_pos
Args#234, required.
const size_t TUNEUP_BUF_SIZE
Central state/object manager and communications hub.
std::string tpt_origin
Addonpart filename.
static std::string getTweakedFlexbodyMedia(TuneupDefPtr &tuneup_entry, FlexbodyID_t flexbody_id, int media_idx, const std::string &orig_val)
static float getTweakedWheelRimRadius(TuneupDefPtr &tuneup_entry, WheelID_t wheel_id, float orig_val)
static Ogre::Vector3 getTweakedFlexbodyRotation(TuneupDefPtr &tuneup_entry, FlexbodyID_t flexbody_id, Ogre::Vector3 orig_val)
std::array< std::string, 2 > twt_media
twt_media[0] Arg#2, required ('wheels[2]': face material, 'meshwheels[2]/flexbodywheels': rim mesh) t...
std::array< std::string, 2 > tpt_media
Media1 = prop mesh; Media2: Steering wheel mesh or beacon flare material.
static Ogre::Vector3 getTweakedFlexbodyOffset(TuneupDefPtr &tuneup_entry, FlexbodyID_t flexbody_id, Ogre::Vector3 orig_val)
std::string tnt_origin
Addonpart filename.
std::set< FlexbodyID_t > protected_flexbodies
Flexbodies which cannot be removed via 'addonpart_tweak_flexbody' or 'addonpart_remove_flexbody' dire...
static std::string getTweakedPropMediaRG(TuneupDefPtr &tuneup_def, PropID_t prop_id, int media_idx, const std::string &orig_val)
bool isPropProtected(PropID_t propid)
static std::string getTweakedWheelMediaRG(TuneupDefPtr &tuneup_def, WheelID_t wheel_id, int media_idx, const std::string &orig_val)
static WheelSide getTweakedWheelSide(TuneupDefPtr &tuneup_entry, WheelID_t wheel_id, WheelSide orig_val)
static float getTweakedWheelTireRadius(TuneupDefPtr &tuneup_entry, WheelID_t wheel_id, float orig_val)
CacheCategoryId category_id
< Data of 'addonpart_tweak_wheel <wheel ID> <media1> <media2> <side flag> <tire radius> <rim radius>'
static Ogre::Vector3 getTweakedNodePosition(TuneupDefPtr &tuneup_entry, NodeNum_t nodenum, Ogre::Vector3 orig_val)
std::map< WheelID_t, WheelSide > force_wheel_sides
UI overrides.
bool isFlexbodyUnwanted(FlexbodyID_t flexbodyid)
WheelSide
Used by rig-def/addonpart/tuneup formats to specify wheel rim mesh orientation.
int FlexbodyID_t
Index to GfxActor::m_flexbodies, use RoR::FLEXBODYID_INVALID as empty value.
Self reference-counting objects, as requred by AngelScript garbage collector.
bool isWheelSideForced(WheelID_t wheelid, WheelSide &out_val) const
static bool isFlexbodyTweaked(TuneupDefPtr &tuneup_entry, FlexbodyID_t flexbody_id, TuneupFlexbodyTweak *&out_tweak)
static void ParseTuneupAttribute(const std::string &line, TuneupDefPtr &tuneup_def)
< Data of 'addonpart_tweak_flexbody <flexbody ID> <offsetX> <offsetY> <offsetZ> <rotX> <rotY> <rotZ> ...
static std::string getTweakedWheelMedia(TuneupDefPtr &tuneup_entry, WheelID_t wheel_id, int media_idx, const std::string &orig_val)
bool isPropForceRemoved(PropID_t propid)
std::map< WheelID_t, TuneupWheelTweak > wheel_tweaks
Mesh name and radius overrides via 'addonpart_tweak_wheel'.
int PropID_t
Index to GfxActor::m_props, use RoR::PROPID_INVALID as empty value.
bool isNodeProtected(NodeNum_t nodenum) const