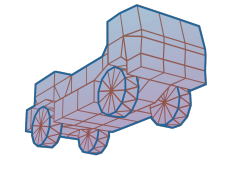 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
25 #ifndef _Hydrax_Hydrax_H_
26 #define _Hydrax_Hydrax_H_
64 Hydrax(Ogre::SceneManager *sm, Ogre::Camera *c, Ogre::Viewport *v);
84 void update(
const Ogre::Real &timeSinceLastFrame);
102 void setModule(
Module::Module* Module,
const bool& DeleteOldModule =
true);
107 void setPolygonMode(
const Ogre::PolygonMode& PM);
117 void setPosition(
const Ogre::Vector3 &Position);
122 void rotate(
const Ogre::Quaternion &q);
130 inline const bool saveCfg(
const Ogre::String &File,
const Ogre::String& Path =
"")
const
132 return mCfgFileManager->save(File, Path);
142 inline const bool loadCfg(
const Ogre::String &File)
const
144 return mCfgFileManager->load(File);
150 void setPlanesError(
const Ogre::Real &PlanesError);
155 void _setStrength(
const Ogre::Real &Strength);
160 void setFullReflectionDistance(
const Ogre::Real &FullReflectionDistance);
162 void setGlobalTransparency(
const Ogre::Real &GlobalTransparencyDistance);
164 void setWaterColor(
const Ogre::Vector3 &WaterColor);
169 void setNormalDistortion(
const Ogre::Real &NormalDistortion);
174 void setSunPosition(
const Ogre::Vector3 &SunPosition);
179 void setSunStrength(
const Ogre::Real &SunStrength);
184 void setSunArea(
const Ogre::Real &SunArea);
189 void setSunColor(
const Ogre::Vector3 &SunColor);
194 void setFoamMaxDistance(
const Ogre::Real &FoamMaxDistance);
199 void setFoamScale(
const Ogre::Real &FoamScale);
204 void setFoamStart(
const Ogre::Real &FoamStart);
209 void setFoamTransparency(
const Ogre::Real &FoamTransparency);
214 void setDepthLimit(
const Ogre::Real &DepthLimit);
219 void setDistLimit(
const Ogre::Real &DistLimit);
225 void setSmoothPower(
const Ogre::Real &SmoothPower);
230 void setCausticsScale(
const Ogre::Real &CausticsScale);
235 void setCausticsPower(
const Ogre::Real &CausticsPower);
240 void setCausticsEnd(
const Ogre::Real &CausticsEnd);
247 mGodRaysExposure = GodRaysExposure;
255 mGodRaysIntensity = GodRaysIntensity;
264 mUnderwaterCameraSwitchDelta = UnderwaterCameraSwitchDelta;
280 void setVisible(
const bool& Visible);
311 return mSceneManager;
327 return mMaterialManager;
343 return mTextureManager;
351 return mGodRaysManager;
359 return mDecalsManager;
367 return mGPUNormalMapManager;
375 return mCfgFileManager;
434 return mModule->getHeigth(Position);
446 return getHeigth(Ogre::Vector2(Position.x, Position.z));
454 return mFullReflectionDistance;
462 return mGlobalTransparency;
486 return mNormalDistortion;
518 return mFoamMaxDistance;
542 return mFoamTransparency;
574 return mCausticsScale;
582 return mCausticsPower;
598 return mGodRaysExposure;
606 return mGodRaysIntensity;
615 return mUnderwaterCameraSwitchDelta;
623 return mCurrentFrameUnderwater;
640 void eventOccurred(
const Ogre::String& eventName,
const Ogre::NameValuePairList *parameters);
649 void _checkVisible();
654 void _checkUnderwater(
const Ogre::Real& timeSinceLastFrame);
void setUnderwaterCameraSwitchDelta(const Ogre::Real &UnderwaterCameraSwitchDelta)
Set the y-displacement under the water needed to change between underwater and overwater mode.
Ogre::Real mNormalDistortion
Normal distortion param.
CfgFileManager * mCfgFileManager
Our Hydrax::CfgFileManager pointer.
GodRaysManager * getGodRaysManager()
Get Hydrax::GodRaysManager.
const Ogre::Real & getSunStrength() const
Get water strength.
const Ogre::Vector3 & getWaterColor() const
Get water color.
Ogre::Real mCausticsEnd
Caustics end.
const Ogre::Vector3 & getPosition() const
Get water position.
Class for manager Normal maps.
const Ogre::Real & getPlanesError() const
Get current clip planes error.
bool mCurrentFrameUnderwater
Is current frame underwater?
Mesh * getMesh()
Get Hydrax::Mesh.
const Ogre::Real & getFoamTransparency() const
Get foam transparency.
Ogre::Real mFoamTransparency
Foam transparency param.
DeviceListener mDeviceListener
Device listener.
MaterialManager * getMaterialManager()
Get Hydrax::MaterialManager.
const Ogre::Vector3 & getSunColor() const
Get sun color.
Ogre::Viewport * mViewport
Pointer to main window viewport.
const bool & isCreated() const
Has create() already called?
Ogre::Vector3 mSunColor
Sun color.
Ogre::Vector3 mGodRaysExposure
God rays exposure factors.
const Ogre::Real & getGlobalTransparency() const
Get global transparency.
const Ogre::Real & getFoamScale() const
Get foam scale.
HydraxComponent mComponents
Hydrax components.
Material/Shader manager class.
void setGodRaysExposure(const Ogre::Vector3 &GodRaysExposure)
Set god rays exposure.
const Ogre::Real & getCausticsEnd() const
Get caustics end.
MaterialManager * mMaterialManager
Our Hydrax::MaterialManager.
TextureManager * mTextureManager
Our Hydrax::TextureManager pointer.
const Ogre::Real & getUnderwaterCameraSwitchDelta() const
Get the y-displacement under the water needed to change between underwater and overwater mode.
Ogre::Real mUnderwaterCameraSwitchDelta
Ogre::Vector3 mSunPosition
Sun position.
Ogre::Real mGlobalTransparency
Global transparency param.
Class to manager GPU normal maps.
const MaterialManager::ShaderMode & getShaderMode() const
Get current shader mode.
RttManager * getRttManager()
Get Hydrax::RttManager.
Ogre::Real mSunArea
Sun area.
Ogre::Real mFoamScale
Foam scale param.
const Ogre::Real & getDepthLimit() const
Get depth limit.
DecalsManager * mDecalsManager
Our Hydrax::DecalsManager pointer.
GPUNormalMapManager * mGPUNormalMapManager
Our Hydrax::GPUNormalMapManager pointer.
Mesh * mMesh
Our Hydrax::Mesh pointer.
Hydrax * mHydrax
Hydrax manager pointer.
const Ogre::Real & getNormalDistortion() const
Get normal distortion.
Module::Module * getModule()
Get our Hydrax::Module::Module.
GPUNormalMapManager * getGPUNormalMapManager()
Get Hydrax::GPUNormalMapManager.
Ogre::Real mFoamStart
Foam start param.
Ogre::Real mFoamMaxDistance
Foam max distance param.
Ogre::Real mGodRaysIntensity
God rays intensity.
GodRaysManager * mGodRaysManager
Our Hydrax::GodRaysManager pointer.
Ogre::Vector3 mWaterColor
Water color param.
Ogre::PolygonMode mPolygonMode
Polygon mode (Solid, Wireframe, Points)
const Ogre::Real & getGodRaysIntensity() const
Get God rays intensity.
Ogre::SceneManager * getSceneManager()
Get scene manager.
Ogre::Camera * getCamera()
Get rendering camera.
const HydraxComponent & getComponents() const
Get hydrax components selected.
const Ogre::PolygonMode & getPolygonMode() const
Get current polygon mode.
CfgFileManager * getCfgFileManager()
Get Hydrax::CfgFileManager.
const Ogre::Real & getFoamStart() const
Get foam start.
Central state/object manager and communications hub.
float getHeigth(const Ogre::Vector3 &Position)
Get the current heigth at a especified world-space point.
Base module class, Override it for create different ways of create water noise.
const bool loadCfg(const Ogre::String &File) const
Load config from file.
Ogre::Viewport * getViewport()
Get main window viewport.
Ogre::Real mCausticsScale
Caustics scale param.
Class wich contains all funtions/variables related to Hydrax water mesh.
Ogre::Vector3 mPosition
Water position.
Ogre::Real mSmoothPower
Smooth power param.
Underwater god rays manager class God rays.
const bool & isVisible() const
Is hydrax water visible?
const Ogre::Real & getSunArea() const
Get sun area.
Class to load/save all Hydrax options from/to a config file.
const Ogre::Real & getFoamMaxDistance() const
Get foam max distance.
MaterialManager::ShaderMode mShaderMode
Current shader mode.
Ogre::Real mDepthLimit
Depth limit param.
const Ogre::Vector3 & getSunPosition() const
Get sun position.
bool mVisible
Is hydrax water visible?
TextureManager * getTextureManager()
Get Hydrax::TextureManager.
const Ogre::Real & getCausticsPower() const
Get caustics power.
const Ogre::Vector3 & getGodRaysExposure() const
Get God rays exposure factors.
HydraxComponent
Hydrax flags to select components wich we want to use.
Module::Module * mModule
Our Hydrax::Module::Module pointer.
Ogre::Real mFullReflectionDistance
Full reflection distance param.
Ogre::Camera * mCamera
Pointer to Ogre::Camera.
const Ogre::Real & getFullReflectionDistance() const
Get full reflection distance.
const bool & _isCurrentFrameUnderwater() const
Is current frame underwater?
Ogre::Real mSunStrength
Sun strength param.
const Ogre::Real & getSmoothPower() const
Get smooth power.
Ogre::Real mDistLimit
Distance limit param (viewable underwater)
RttManager * mRttManager
Our Hydrax::RttManager.
const Ogre::Real & getDistLimit() const
Get distance limit (viewable underwater)
bool mCreated
Has create() already called?
const Ogre::Real & getCausticsScale() const
Get caustics scale.
Ogre::Real mPlanesError
Planes error, y axis clipplanes displacement.
void setGodRaysIntensity(const Ogre::Real &GodRaysIntensity)
Set god rays intensity.
float getHeigth(const Ogre::Vector2 &Position)
Get the current heigth at a especified world-space point.
Ogre::SceneManager * mSceneManager
Pointer to Ogre::SceneManager.
Ogre::Real mCausticsPower
Caustics power.
const bool saveCfg(const Ogre::String &File, const Ogre::String &Path="") const
Save hydrax config to file.
DecalsManager * getDecalsManager()
Get Hydrax::DecalsManager.