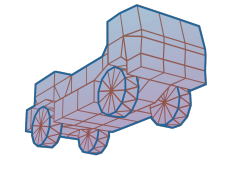 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
40 :m_silent_mode(silent_mode)
56 Ogre::DataStreamPtr datastream = Ogre::ResourceGroupManager::getSingleton().openResource(entry->
fname, entry->
resource_group);
66 Keyword block = Keyword::INVALID;
82 keyword = Keyword::SET_MANAGEDMATERIALS_OPTIONS;
84 if (
keyword != Keyword::INVALID)
86 if (
keyword == Keyword::SET_MANAGEDMATERIALS_OPTIONS)
92 else if (keyword == Keyword::MANAGEDMATERIALS
94 ||
keyword == Keyword::FLEXBODIES
123 catch (Ogre::Exception& e)
127 fmt::format(
"Could not use addonpart: Error parsing file '{}', message: {}",
128 entry->
fname, e.getFullDescription()));
145 Ogre::DataStreamPtr datastream = Ogre::ResourceGroupManager::getSingleton().openResource(addonpart_entry->
fname, addonpart_entry->
resource_group);
184 catch (Ogre::Exception& e)
188 fmt::format(
"Addonpart unwanted elements check: Error parsing file '{}', message: {}",
189 addonpart_entry->
fname, e.getFullDescription()));
224 if (str ==
"mesh_standard") def.
type = ManagedMaterialType::MESH_STANDARD;
225 if (str ==
"mesh_transparent") def.
type = ManagedMaterialType::MESH_TRANSPARENT;
226 if (str ==
"flexmesh_standard") def.
type = ManagedMaterialType::FLEXMESH_STANDARD;
227 if (str ==
"flexmesh_transparent") def.
type = ManagedMaterialType::FLEXMESH_TRANSPARENT;
240 m_module->managedmaterials.push_back(def);
260 fmt::format(
"Error parsing addonpart file '{}': 'install_prop' has only {} arguments, expected {}",
265 int importflags = Node::Ref::REGULAR_STATE_IS_VALID | Node::Ref::REGULAR_STATE_IS_NUMBERED;
283 case SpecialProp::BEACON:
294 case SpecialProp::DASHBOARD_LEFT:
295 case SpecialProp::DASHBOARD_RIGHT:
330 int importflags = Node::Ref::REGULAR_STATE_IS_VALID | Node::Ref::REGULAR_STATE_IS_NUMBERED;
360 for (
unsigned int i = range.
start.
Num(); i <= range.
end.
Num(); ++i)
362 Node::Ref ref(
"", i, Node::Ref::REGULAR_STATE_IS_VALID | Node::Ref::REGULAR_STATE_IS_NUMBERED, 0);
367 m_module->flexbodies.push_back(def);
382 int importflags = Node::Ref::REGULAR_STATE_IS_VALID | Node::Ref::REGULAR_STATE_IS_NUMBERED;
420 int importflags = Node::Ref::REGULAR_STATE_IS_VALID | Node::Ref::REGULAR_STATE_IS_NUMBERED;
458 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': marking prop '{}' as UNWANTED",
463 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': skipping prop '{}' because it's marked PROTECTED",
482 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': marking flexbody '{}' as UNWANTED",
487 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': skipping flexbody '{}' because it's marked PROTECTED",
506 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': marking flare '{}' as UNWANTED",
511 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': skipping flare '{}' because it's marked PROTECTED",
530 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': marking exhaust '{}' as UNWANTED",
535 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': skipping exhaust '{}' because it's marked PROTECTED",
555 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': marking managedmaterial '{}' as UNWANTED",
560 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': skipping managedmaterial '{}' because it's marked PROTECTED",
592 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': Sheduling tweak for wheel '{}'"
593 " with params {{ media1={}, media2={}, side={}, tire_radius={}, rim_radius={} }}",
599 this->
Log(
fmt::format(
"[RoR|Addonpart] WARNING: file '{}', directive '{}': Resetting tweaks for wheel '{}' due to conflict with '{}'",
608 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': skipping wheel '{}' because it's marked PROTECTED",
637 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': Scheduling tweak for node '{}'"
638 " with params {{ x={}, y={}, z={} }}",
644 this->
Log(
fmt::format(
"[RoR|Addonpart] WARNING: file '{}', directive '{}': Resetting tweaks for node '{}' due to conflict with '{}'",
653 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': skipping node '{}' because it's marked PROTECTED",
682 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': Scheduling tweak for cinecam '{}'"
683 " with params {{ x={}, y={}, z={} }}",
689 this->
Log(
fmt::format(
"[RoR|Addonpart] WARNING: file '{}', directive '{}': Resetting tweaks for cinecam '{}' due to conflict with '{}'",
698 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': skipping cinecam '{}' because it's marked PROTECTED",
735 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': Scheduling tweak for flexbody '{}'"
736 " with params {{ offsetX={}, offsetY={}, offsetZ={}, rotX={}, rotY={}, rotZ={}, media={} }}",
743 this->
Log(
fmt::format(
"[RoR|Addonpart] WARNING: file '{}', directive '{}': Resetting tweaks for flexbody '{}' due to conflict with '{}'",
752 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': skipping flexbody '{}' because it's marked PROTECTED",
791 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': Scheduling tweak for prop '{}'"
792 " with params {{ media1={}, offsetX={}, offsetY={}, offsetZ={}, rotX={}, rotY={}, rotZ={}, media2={} }}",
800 this->
Log(
fmt::format(
"[RoR|Addonpart] WARNING: file '{}', directive '{}': Resetting tweaks for prop '{}' due to conflict with '{}'",
809 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': skipping prop '{}' because it's marked PROTECTED",
840 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': Scheduling tweak for managed material '{}'"
841 " with params {{ type={}, media1={}, media2={}, media3={} }}",
846 this->
Log(
fmt::format(
"[RoR|Addonpart] WARNING: file '{}', directive '{}': Resetting tweaks for managed material '{}' due to conflict with '{}'",
855 this->
Log(
fmt::format(
"[RoR|Addonpart] INFO: file '{}', directive '{}': skipping managed material '{}' because it's marked PROTECTED",
863 LOG(
fmt::format(
"[RoR|Addonpart] -- Performing `RecordAddonpartConflicts()` between '{}' and '{}' ~ this involves generating dummy tuneups (hence messages below) --", addonpart1->
fname, addonpart2->
fname));
885 NodeNum_t suspect = i_pair.second.tnt_nodenum;
889 conflicts.push_back(
AddonPartConflict{addonpart1, addonpart2,
"addonpart_tweak_node", (int)suspect});
890 LOG(
fmt::format(
"[RoR|Addonpart] Found conflict between '{}' and '{}' - node {} is tweaked by both", addonpart1->
fname, addonpart2->
fname, (
int)suspect));
897 WheelID_t suspect = i_pair.second.twt_wheel_id;
901 conflicts.push_back(
AddonPartConflict{addonpart1, addonpart2,
"addonpart_tweak_wheel", (int)suspect});
902 LOG(
fmt::format(
"[RoR|Addonpart] Found conflict between '{}' and '{}' - wheel {} is tweaked by both", addonpart1->
fname, addonpart2->
fname, (
int)suspect));
909 PropID_t suspect = i_pair.second.tpt_prop_id;
913 conflicts.push_back(
AddonPartConflict{addonpart1, addonpart2,
"addonpart_tweak_prop", (int)suspect});
914 LOG(
fmt::format(
"[RoR|Addonpart] Found conflict between '{}' and '{}' - prop {} is tweaked by both", addonpart1->
fname, addonpart2->
fname, (
int)suspect));
925 conflicts.push_back(
AddonPartConflict{addonpart1, addonpart2,
"addonpart_tweak_flexbody", (int)suspect});
926 LOG(
fmt::format(
"[RoR|Addonpart] Found conflict between '{}' and '{}' - flexbody {} is tweaked by both", addonpart1->
fname, addonpart2->
fname, (
int)suspect));
930 LOG(
fmt::format(
"[RoR|Addonpart] -- Done with `RecordAddonpartConflicts()` between '{}' and '{}' --", addonpart1->
fname, addonpart2->
fname));
935 if (!addonpart1 || !addonpart2)
942 if ((conflict.atc_addonpart1 == addonpart1 && conflict.atc_addonpart2 == addonpart2) ||
943 (conflict.atc_addonpart1 == addonpart2 && conflict.atc_addonpart2 == addonpart1))
971 if (conflicts.size() > 0)
976 dialog->
mbc_title =
_LC(
"Tuning",
"Cannot install addon part, conflicts were detected.");
981 for (
size_t i=0; i < conflicts.size(); i++)
985 i+1, conflicts.size(),
986 conflicts[i].atc_addonpart2->dname, conflicts[i].atc_addonpart2->fname,
987 conflicts[i].atc_keyword, conflicts[i].atc_element_id);
999 return conflicts.size() > 0;
std::vector< MessageBoxButton > mbc_buttons
#define ROR_ASSERT(_EXPR)
Game state manager and message-queue provider.
void ResolveUnwantedAndTweakedElements(TuneupDefPtr &tuneup, CacheEntryPtr &addonpart_entry)
Evaluates 'addonpart_unwanted_*' elements, respecting 'protected_*' directives in the tuneup.
std::shared_ptr< RigDef::Document::Module > m_module
@ MSG_GUI_SHOW_MESSAGE_BOX_REQUESTED
Payload = MessageBoxConfig* (owner)
GenericDocumentPtr m_document
std::string twt_origin
Addonpart filename.
Ogre::String dname
name parsed from the file
Legacy parser resolved references on-the-fly and the condition to check named nodes was "are there an...
NodeNum_t tnt_nodenum
Arg#1, required.
bool isTokInt(int offset=0) const
static bool isWheelTweaked(TuneupDefPtr &tuneup_entry, WheelID_t wheel_id, TuneupWheelTweak *&out_tweak)
bool isManagedMatProtected(const std::string &matname) const
float twt_tire_radius
Arg#5, optional.
std::set< PropID_t > unwanted_props
'addonpart_unwanted_prop' directives.
< Data of 'addonpart_tweak_prop <prop ID> <offsetX> <offsetY> <offsetZ> <rotX> <rotY> <rotZ> <media1>...
float twt_rim_radius
Arg#6, optional, only applies to some wheel types.
Ogre::Vector3 tft_rotation
float getTokNumeric(int offset=0) const
bool isTokKeyword(int offset=0) const
Generic UI dialog (not modal). Invocable from scripting. Any number of buttons. Configurable to fire ...
void ProcessUnwantedFlare()
int CineCameraID_t
Index into Actor::ar_cinecam_node and Actor::ar_camera_node_* arrays; use RoR::CINECAMERAID_INVALID a...
static bool isPropTweaked(TuneupDefPtr &tuneup_entry, PropID_t flexbody_id, TuneupPropTweak *&out_tweak)
void ProcessUnwantedExhaust()
std::string tft_origin
Addonpart filename.
std::string tmt_type
Arg#2, required.
std::set< std::string > unwanted_managedmats
'addonpart_unwanted_managedmaterial' directives.
Ogre::String damaged_diffuse_map
std::map< PropID_t, TuneupPropTweak > prop_tweaks
Mesh name(s), offset and rotation overrides via 'addonpart_tweak_prop'.
Truck file format(technical spec)
std::set< FlexbodyID_t > unwanted_flexbodies
'addonpart_unwanted_flexbody' directives.
static const BitMask_t OPTION_ALLOW_SLASH_COMMENTS
Allow comments starting with //.
bool isTokLineBreak(int offset=0) const
GenericDocContextPtr m_context
std::string getTokString(int offset=0) const
void ProcessUnwantedManagedMat()
bool isFlexbodyProtected(FlexbodyID_t flexbodyid) const
void putMessage(MessageArea area, MessageType type, std::string const &msg, std::string icon="")
bool isTokFloat(int offset=0) const
std::shared_ptr< RigDef::Document::Module > TransformToRigDefModule(CacheEntryPtr &addonpart_entry)
transforms the addonpart to RigDef::File::Module (fake 'section/end_section') used for spawning.
Ogre::String resource_group
Resource group of the loaded bundle. Empty if not loaded yet.
bool m_silent_mode
To block logging during conflict resolution (which works by generating dummy tuneups - would confuse ...
std::set< std::string > use_addonparts
Addonpart filenames.
float getTokFloat(int offset=0) const
TuneupDefPtr addonpart_data_only
Cached addonpart data (dummy tuneup), only used for evaluating conflicts, see AddonPartUtility::Recor...
Ogre::Vector3 tpt_rotation
std::vector< AddonPartConflict > AddonPartConflictVec
void ProcessManagedMaterial()
int ExhaustID_t
Index into GfxActor::m_exhausts, use RoR::EXHAUSTID_INVALID as empty value.
WheelID_t twt_wheel_id
Arg#1, required.
bool isPropProtected(PropID_t propid) const
bool isTokString(int offset=0) const
void ProcessDirectiveSetManagedMaterialsOptions()
CineCameraID_t tct_cinecam_id
Arg#1, required.
static bool isNodeTweaked(TuneupDefPtr &tuneup_entry, NodeNum_t nodenum, TuneupNodeTweak *&out_tweak)
< Data of 'addonpart_tweak_cinecam <cinecam ID> <posX> <posY> <posZ>'
FlexbodyID_t tft_flexbody_id
ManagedMaterialsOptions options
void ProcessUnwantedProp()
static void ResetUnwantedAndTweakedElements(TuneupDefPtr &tuneup)
std::map< std::string, TuneupManagedMatTweak > managedmat_tweaks
Managed material overrides via 'addonpart_tweak_managedmaterial'.
Ogre::String material_name
std::map< NodeNum_t, TuneupNodeTweak > node_tweaks
Node position overrides via 'addonpart_tweak_node'.
std::set< FlareID_t > unwanted_flares
'addonpart_unwanted_flare' directives.
< Data of 'addonpart_tweak_managedmaterial <name> <type> <media1> <media2> [<media3>]'
std::string dashboard_link
Only 'd' type flares.
std::string tmt_origin
Addonpart filename.
uint16_t NodeNum_t
Node position within Actor::ar_nodes; use RoR::NODENUM_INVALID as empty value.
CacheEntryPtr FindEntryByFilename(RoR::LoaderType type, bool partial, const std::string &_filename_maybe_bundlequalified)
Returns NULL if none found; "Bundle-qualified" format also specifies the ZIP/directory in modcache,...
< Data of 'addonpart_tweak_node <nodenum> <posX> <posY> <posZ>'
static void RecordAddonpartConflicts(CacheEntryPtr addonpart1, CacheEntryPtr addonpart2, AddonPartConflictVec &conflicts)
A database of user-installed content alias 'mods' (vehicles, terrains...)
static SpecialProp IdentifySpecialProp(const std::string &str)
void ProcessTweakCineCamera()
WheelSide twt_side
Arg#4, optional, default LEFT (Only applicable to mesh/flexbody wheels)
bool isTokNumeric(int offset=0) const
std::map< FlexbodyID_t, TuneupFlexbodyTweak > flexbody_tweaks
Mesh name, offset and rotation overrides via 'addonpart_tweak_flexbody'.
void PushMessage(Message m)
Doesn't guarantee order! Use ChainMessage() if order matters.
int WheelID_t
Index to Actor::ar_wheels, use RoR::WHEELID_INVALID as empty value.
Ogre::String specular_map
Ogre::Vector3 tnt_pos
Args#234, required.
CacheEntryPtr m_addonpart_entry
bool isWheelProtected(WheelID_t wheelid) const
std::vector< Node::Range > node_list_to_import
Central state/object manager and communications hub.
std::string tpt_origin
Addonpart filename.
< Conflict between two addonparts tweaking the same element
static bool DoubleCheckForAddonpartConflict(ActorPtr target_actor, CacheEntryPtr addonpart_entry)
GameContext * GetGameContext()
std::set< ExhaustID_t > unwanted_exhausts
'addonpart_unwanted_exhaust' directives.
void Log(const std::string &text)
const char * getStringData(int offset=0) const
bool endOfFile(int offset=0) const
void ProcessTweakFlexbody()
static bool CheckForAddonpartConflict(CacheEntryPtr addonpart1, CacheEntryPtr addonpart2, AddonPartConflictVec &conflicts)
std::array< std::string, 2 > twt_media
twt_media[0] Arg#2, required ('wheels[2]': face material, 'meshwheels[2]/flexbodywheels': rim mesh) t...
float getFloatData(int offset=0) const
CacheSystem * GetCacheSystem()
std::array< std::string, 2 > tpt_media
Media1 = prop mesh; Media2: Steering wheel mesh or beacon flare material.
std::vector< Node::Ref > node_list
std::string tnt_origin
Addonpart filename.
void LoadResource(CacheEntryPtr &t)
Loads the associated resource bundle if not already done.
Unified game event system - all requests and state changes are reported using a message.
std::map< CineCameraID_t, TuneupCineCameraTweak > cinecam_tweaks
Cinecam position overrides via 'addonpart_tweak_cinecam'.
RigDef::ManagedMaterialsOptions m_managedmaterials_options
Checks the rig-def file syntax and loads data to memory.
int FlareID_t
Index into Actor::ar_flares, use RoR::FLAREID_INVALID as empty value.
bool isFlareProtected(FlareID_t flareid) const
std::string getTokKeyword(int offset=0) const
DashboardSpecial special_prop_dashboard
std::string tmt_name
Arg#1, required.
< Data of 'addonpart_tweak_wheel <wheel ID> <media1> <media2> <side flag> <tire radius> <rim radius>'
float mbc_content_width
Parameter to ImGui::SetContentWidth() - hard limit on content size.
int control_number
Only 'u' type flares.
void ProcessUnwantedFlexbody()
@ CONSOLE_MSGTYPE_ACTOR
Parsing/spawn/simulation messages for actors.
virtual void loadFromDataStream(Ogre::DataStreamPtr datastream, BitMask_t options=0)
bool isExhaustProtected(ExhaustID_t exhaustid) const
AddonPartUtility(bool silent_mode=false)
NOTE: Modcache processes this format directly using RoR::GenericDocument, see RoR::CacheSystem::FillA...
bool isCineCameraProtected(CineCameraID_t cinecamid) const
int FlexbodyID_t
Index to GfxActor::m_flexbodies, use RoR::FLEXBODYID_INVALID as empty value.
std::string tct_origin
Addonpart filename.
static bool isFlexbodyTweaked(TuneupDefPtr &tuneup_entry, FlexbodyID_t flexbody_id, TuneupFlexbodyTweak *&out_tweak)
bool isTokComment(int offset=0) const
@ MSG_GUI_HIDE_MESSAGE_BOX_REQUESTED
< Data of 'addonpart_tweak_flexbody <flexbody ID> <offsetX> <offsetY> <offsetZ> <rotX> <rotY> <rotZ> ...
static const BitMask_t OPTION_ALLOW_NAKED_STRINGS
Allow strings without quotes, for backwards compatibility.
TuneupDefPtr & getWorkingTuneupDef()
std::array< std::string, 3 > tmt_media
Arg#3, required, Arg#4, optional, Arg#5, optional.
std::map< WheelID_t, TuneupWheelTweak > wheel_tweaks
Mesh name and radius overrides via 'addonpart_tweak_wheel'.
BeaconSpecial special_prop_beacon
int PropID_t
Index to GfxActor::m_props, use RoR::PROPID_INVALID as empty value.
Ogre::Vector3 tct_pos
Args#234, required.
bool isNodeProtected(NodeNum_t nodenum) const
Ogre::String flare_material_name
void ProcessTweakManagedMat()
Ogre::String fname
filename
int getTokInt(int offset=0) const