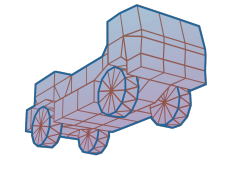 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
66 std::vector<CabTexcoord>& texcoords,
69 std::vector<CabSubmesh>& submeshes,
Ogre::Vector3 UpdateMesh()
BackmeshType backmesh_type
unsigned short * m_indices
Ogre::HardwareVertexBufferSharedPtr m_hw_vbuf
A visual mesh, forming a chassis for softbody actor At most one instance is created per actor.
FlexObjVertex * m_vertices
std::vector< Ogre::SubMesh * > m_submeshes
Core data structures for simulation; Everything affected by by either physics, network or user intera...
Central state/object manager and communications hub.
Physics: A vertex in the softbody structure.
int ComputeVertexPos(int tidx, int v, std::vector< CabSubmesh > &submeshes)
Compute vertex position in the vertexbuffer (0-based offset) for node v of triangle tidx
FlexObj(RoR::GfxActor *gfx_actor, node_t *all_nodes, std::vector< CabTexcoord > &texcoords, int numtriangles, int *triangles, std::vector< CabSubmesh > &submeshes, char *texname, const char *name, char *backtexname, char *transtexname)
Submesh for old-style actor body (the "cab")
void ScaleFlexObj(float factor)
Texture coordinates for old-style actor body (the "cab")
RoR::GfxActor * m_gfx_actor
Ogre::Vector3 UpdateFlexObj()
Ogre::VertexDeclaration * m_vertex_format