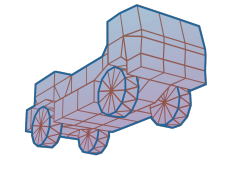 |
RigsofRods
Soft-body Physics Simulation
|
Go to the documentation of this file.
62 GravityCmd():
ConsoleCmd(
"gravity",
"[<number> or <constant>]",
_L(
"Get or set terrain gravity. Constants: earth/moon/mars/jupiter.")) {}
64 void Run(Ogre::StringVector
const& args)
override
94 void Run(Ogre::StringVector
const& args)
override
101 reply << m_name <<
": ";
105 reply <<
_L(
"This terrain does not have water.");
128 void Run(Ogre::StringVector
const& args)
override
136 reply << m_name <<
": ";
150 reply <<
_L(
"Terrain height at position: ")
151 <<
"x: " << pos.x <<
" z: " << pos.z <<
" = "
162 void Run(Ogre::StringVector
const& args)
override
171 reply << m_name <<
": ";
176 if (args.size() == 1)
179 reply <<
_L(
"Missing parameter: ") << m_usage;
184 if (
App::GetGameContext()->GetTerrain()->getObjectManager()->LoadTerrainObject(args[1], pos, Ogre::Vector3::ZERO,
"Console",
""))
187 reply <<
_L(
"Spawned object at position: ") <<
"x: " << pos.x <<
" z: " << pos.z;
192 reply <<
_L(
"Could not spawn object");
196 catch (std::exception& e)
210 void Run(Ogre::StringVector
const& args)
override
213 reply << m_name <<
": ";
218 const char* msg = (now_logging) ?
" logging to console enabled" :
" logging to console disabled";
231 void Run(Ogre::StringVector
const& args)
override
234 reply << m_name <<
": ";
250 void Run(Ogre::StringVector
const& args)
override
256 reply << m_name <<
": ";
263 reply <<
_L(
"Character position: ") <<
"x: " << pos.x <<
" y: " << pos.y <<
" z: " << pos.z;
268 reply <<
_L(
"Vehicle position: ") <<
"x: " << pos.x <<
" y: " << pos.y <<
" z: " << pos.z;
280 void Run(Ogre::StringVector
const& args)
override
286 reply << m_name <<
": ";
289 if (args.size() != 4)
292 reply <<
_L(
"usage: goto x y z");
303 reply <<
_L(
"Character position set to: ") <<
"x: " << pos.x <<
" y: " << pos.y <<
" z: " << pos.z;
309 reply <<
_L(
"Vehicle position set to: ") <<
"x: " << pos.x <<
" y: " << pos.y <<
" z: " << pos.z;
323 void Run(Ogre::StringVector
const& args)
override
329 reply << m_name <<
": ";
332 #ifdef USE_ANGELSCRIPT
338 for (
int i = 1; i < args.size(); ++i)
340 code <<
" " << args[i];
345 reply <<
" >>> " << code.
ToCStr();
352 reply <<
_L(
"Scripting disabled in this build");
363 void Run(Ogre::StringVector
const& args)
override
374 void Run(Ogre::StringVector
const& args)
override
382 line.
Clear() << cmd_pair.second->getName() <<
" "
383 << cmd_pair.second->GetUsage() <<
" - " << cmd_pair.second->GetDoc();
401 void Run(Ogre::StringVector
const& args)
override
404 reply << m_name <<
": ";
407 #ifdef USE_ANGELSCRIPT
408 if (args.size() == 1)
411 reply <<
_L(
"Missing parameter: ") << m_usage;
419 reply <<
_L(
"Failed to load script. See 'Angelscript.log' or use console command `log` and retry.");
424 reply <<
fmt::format(
_L(
"Script '{}' running with ID '{}'"), args[1],
id);
429 reply <<
_L(
"Scripting disabled in this build");
444 void Run(Ogre::StringVector
const& args)
override
448 bool match = args.size() == 1;
449 for (
size_t i = 1; i < args.size(); ++i)
451 if (pair.first.find(args[i]) != std::string::npos)
461 reply <<
"vars: " << pair.first <<
"=" << pair.second->getStr() <<
" (";
464 else if (pair.second->hasFlag(
CVAR_TYPE_INT)) { reply <<
"int"; }
466 else { reply <<
"string"; }
468 if (pair.second->hasFlag(
CVAR_ARCHIVE)) { reply <<
", archive"; }
469 if (pair.second->hasFlag(
CVAR_NO_LOG)) { reply <<
", no log"; }
481 SetCmd():
ConsoleCmd(
"set",
"<cvar> [<flags>] [<value>]",
_L(
"Get or set value of existing CVar")) {}
483 void Run(Ogre::StringVector
const& args)
override
486 reply << m_name <<
": ";
489 if (args.size() == 1)
492 reply << this->GetUsage() <<
" - " << this->GetDoc();
509 reply <<
_L(
"No such CVar: ") << args[1];
520 SetCVarCmd(std::string
const& name, std::string
const& usage, std::string
const& doc,
int flag):
521 ConsoleCmd(name, usage, doc), m_cvar_flag(flag)
524 void Run(Ogre::StringVector
const& args)
override
527 reply << m_name <<
": ";
530 if (args.size() == 1)
533 reply << this->GetUsage() <<
" - " << this->GetDoc() <<
"Switches: --autoapply/--allowstore/--autostore";
537 int flags = m_cvar_flag;
539 for (i = 1; i < args.size(); ++i)
545 if (i == args.size())
548 reply << this->GetUsage() <<
" - " << this->GetDoc() <<
"Switches: --archive";
553 if (args.size() > (i+1))
595 ClearCmd():
ConsoleCmd(
"clear",
"[<type>]",
_L(
"Clear console history. Types: all/info/net/chat/terrn/actor/script")) {}
597 void Run(Ogre::StringVector
const& args)
override
599 if (args.size() < 2 || args[1] ==
"all")
608 if (args[1] ==
"chat")
612 else if (args[1] ==
"net")
638 auto erase_begin = std::remove_if(lock.
messages.begin(), lock.
messages.end(), filter_fn);
680 if (msg[0] ==
'/' || msg[0] ==
'\\')
683 _L(
"Using slashes before commands are deprecated, you can now type command without any slashes"));
684 msg.erase(msg.begin());
694 Ogre::StringVector args = Ogre::StringUtil::split(msg,
" ");
699 found->second->Run(args);
713 reply <<
_L(
"unknown command: ") << msg;
729 reply <<
_L(
"Only allowed when simulation is running");
733 reply <<
_L(
"Not allowed in current app state");
#define ROR_ASSERT(_EXPR)
Game state manager and message-queue provider.
@ CVAR_NO_LOG
Will not be written to RoR.log.
virtual float GetStaticWaterHeight()=0
Returns static water level configured in 'terrn2'.
void resetPosition(Ogre::Vector3 translation, bool setInitPosition)
Moves the actor to given world coords.
void setPosition(Ogre::Vector3 position)
CVar * cVarGet(std::string const &input_name, int flags)
Get cvar by short/long name, or create new one using input as short name.
void Run(Ogre::StringVector const &args) override
@ SE_ANGELSCRIPT_MANIPULATIONS
triggered when the user tries to dynamically use the scripting capabilities (prevent cheating) args: ...
void Run(Ogre::StringVector const &args) override
@ CONSOLE_MSGTYPE_TERRN
Parsing/spawn/simulation messages for terrain.
void Run(Ogre::StringVector const &args) override
ActorInstanceID_t ar_instance_id
Static attr; session-unique ID.
std::string const & getName() const
Truck file format(technical spec)
SetCVarCmd(std::string const &name, std::string const &usage, std::string const &doc, int flag)
Character * GetPlayerCharacter()
void setGravity(float value)
void TRIGGER_EVENT_ASYNC(scriptEvents type, int arg1, int arg2ex=0, int arg3ex=0, int arg4ex=0, std::string arg5ex="", std::string arg6ex="", std::string arg7ex="", std::string arg8ex="")
Asynchronously (via MSG_SIM_SCRIPT_EVENT_TRIGGERED) invoke script function eventCallbackEx(),...
< TODO: Mixed gfx+physics (waves) - must be separated ~ only_a_ptr, 02/2018
CVar * cVarFind(std::string const &input_name)
Find cvar by short/long name.
void Run(Ogre::StringVector const &args) override
void Run(Ogre::StringVector const &args) override
void Run(Ogre::StringVector const &args) override
int executeString(Ogre::String command)
executes a string (useful for the console)
void putMessage(MessageArea area, MessageType type, std::string const &msg, std::string icon="")
void Run(Ogre::StringVector const &args) override
ScriptUnitId_t loadScript(Ogre::String scriptname, ScriptCategory category=ScriptCategory::TERRAIN, ActorPtr associatedActor=nullptr, std::string buffer="")
Loads a script.
@ CVAR_ARCHIVE
Will be written to RoR.cfg.
std::vector< Message > & messages
ScriptEngine * GetScriptEngine()
void Run(Ogre::StringVector const &args) override
float GetHeightAt(float x, float z)
virtual void UpdateWater()=0
float getWaterHeight() const
static const ScriptUnitId_t SCRIPTUNITID_INVALID
std::string const & getStr() const
void cVarAssign(CVar *cvar, std::string const &value)
Parse value by cvar type.
@ ASMANIP_CONSOLE_SNIPPET_EXECUTED
void Run(Ogre::StringVector const &args) override
void Run(Ogre::StringVector const &args) override
void PushMessage(Message m)
Doesn't guarantee order! Use ChainMessage() if order matters.
int ScriptUnitId_t
Unique sequentially generated ID of a loaded and running scriptin session. Use ScriptEngine::getScrip...
void BroadcastChatMsg(const char *msg)
const char * ToCStr() const
Ogre::Vector3 getPosition()
Central state/object manager and communications hub.
GameContext * GetGameContext()
void Run(Ogre::StringVector const &args) override
void doCommand(std::string msg)
Identify and execute any console line.
Ogre::Vector3 getPosition()
void triggerEvent(scriptEvents eventnum, int arg1=0, int arg2ex=0, int arg3ex=0, int arg4ex=0, std::string arg5ex="", std::string arg6ex="", std::string arg7ex="", std::string arg8ex="")
triggers an event; Not to be used by the end-user.
@ CONSOLE_SYSTEM_REPLY
Success.
const char *const ROR_VERSION_STRING
Quake-style console variable, defined in RoR.cfg or crated via Console UI and scripts.
CVar * diag_log_console_echo
bool CheckAppState(AppState state)
std::string const & getName() const
Base (abstract) console command.
Unified game event system - all requests and state changes are reported using a message.
@ SE_TRUCK_TELEPORT
triggered when the user teleports the truck, the argument refers to the actor ID of the vehicle
void regBuiltinCommands()
Register builtin commands.
void Run(Ogre::StringVector const &args) override
@ CONSOLE_MSGTYPE_ACTOR
Parsing/spawn/simulation messages for actors.
static const float DEFAULT_GRAVITY
earth gravity
@ CONSOLE_MSGTYPE_INFO
Generic message.
@ CONSOLE_MSGTYPE_SCRIPT
Messages sent from scripts.
virtual void SetStaticWaterHeight(float value)=0
void Run(Ogre::StringVector const &args) override
const char *const ROR_BUILD_DATE
const ActorPtr & GetPlayerActor()
void Run(Ogre::StringVector const &args) override
const char *const ROR_BUILD_TIME
void Run(Ogre::StringVector const &args) override
@ CUSTOM
Loaded by user via either: A) ingame console 'loadscript'; B) RoR.cfg 'app_custom_scripts'; C) comman...
@ MSG_APP_SHUTDOWN_REQUESTED
void Run(Ogre::StringVector const &args) override
const TerrainPtr & GetTerrain()